Gleam for backend development
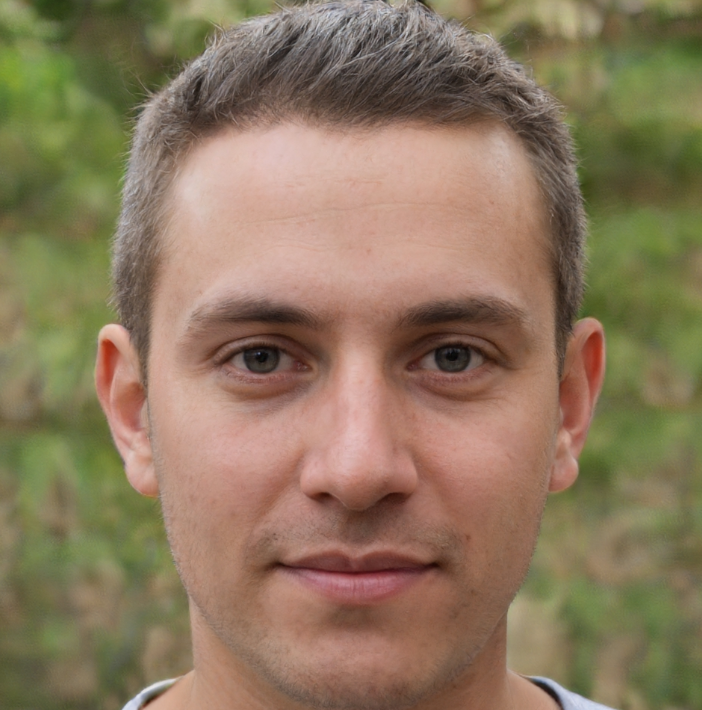
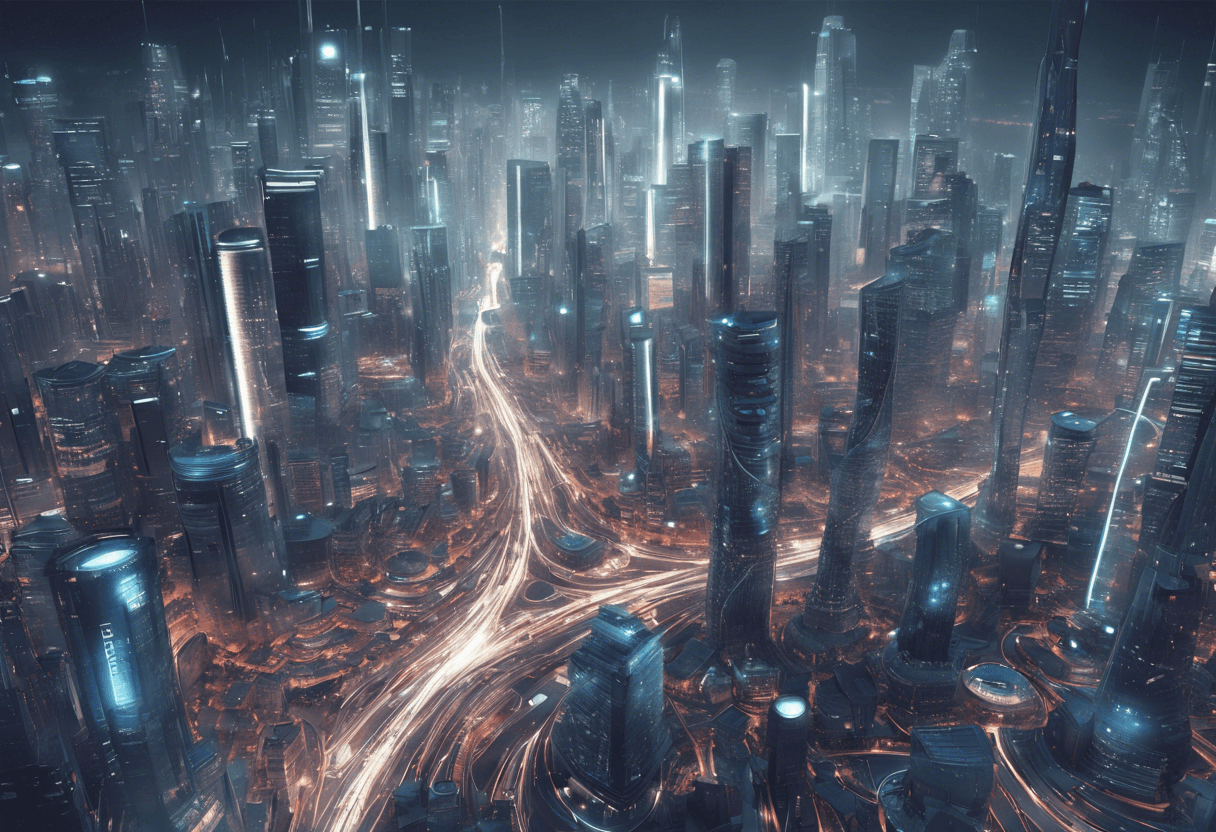
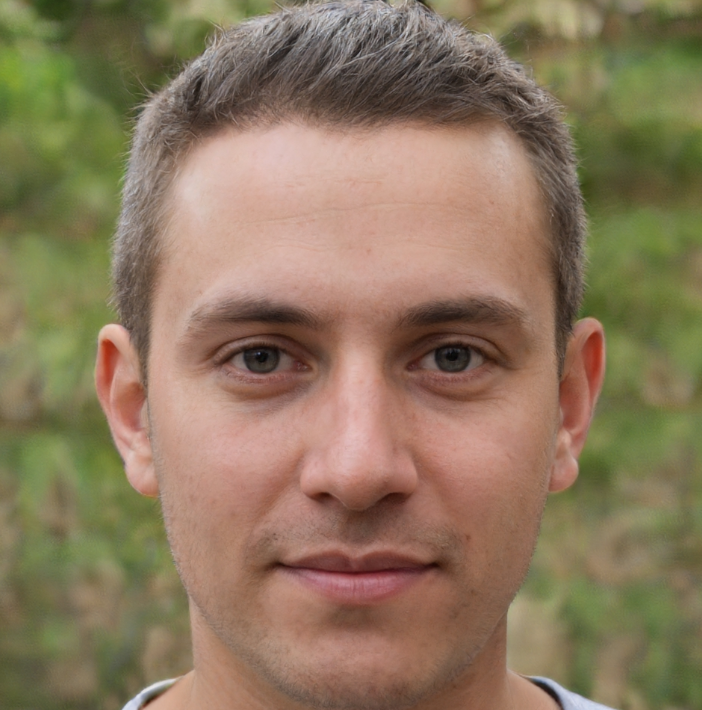
Gleam: The Future of Backend Development
The world of backend development is constantly evolving, with new technologies and frameworks emerging every year. One such technology that has been gaining traction recently is Gleam. In this article, we'll explore what Gleam is, its features, and how it's changing the face of backend development.
What is Gleam?
Gleam is a statically typed, compiled language that's designed specifically for building scalable and efficient backend applications. It's a relatively new language, first released in 2020, but it has already gained a significant following among developers. Gleam is designed to be a more efficient and safer alternative to languages like Node.js and Python, which are commonly used for backend development.
Gleam's Design Principles
Gleam is built around several key design principles that make it an attractive choice for backend development. Firstly, Gleam is statically typed, which means that the type system checks the code at compile time, rather than at runtime. This eliminates the possibility of type-related errors at runtime, making the code more reliable and efficient.
Secondly, Gleam is compiled to machine code, which means that it doesn't require a runtime environment to execute. This makes Gleam applications faster and more lightweight than applications built with interpreted languages like Node.js.
Lastly, Gleam has a strong focus on concurrency and parallelism. The language is designed to make it easy to write concurrent code, which is essential for building scalable and efficient backend applications.
Gleam's Syntax and Features
Gleam's syntax is similar to languages like Rust and Swift, with a focus on simplicity and readability. The language has a number of features that make it well-suited for backend development, including:
- Type inference: Gleam's type system can automatically infer the types of variables, eliminating the need for explicit type annotations.
- Pattern matching: Gleam's pattern matching system allows developers to write concise and expressive code that's easy to read and maintain.
- Concurrency: Gleam has built-in support for concurrency and parallelism, making it easy to write efficient and scalable code.
Gleam's Ecosystem
Gleam's ecosystem is still evolving, but it already has a number of libraries and frameworks that make it easy to build backend applications. Some notable examples include:
- Gleam Framework: A lightweight web framework that makes it easy to build RESTful APIs and web applications.
- Gleam DB: A database library that provides a simple and efficient way to interact with databases.
- Gleam Auth: An authentication library that provides a simple and secure way to manage user authentication.
Use Cases for Gleam
Gleam is a versatile language that can be used for a wide range of backend development tasks. Some potential use cases for Gleam include:
- Web development: Gleam's lightweight web framework and efficient concurrency model make it an attractive choice for building scalable web applications.
- Microservices: Gleam's focus on concurrency and parallelism make it well-suited for building microservices that need to handle high volumes of traffic.
- Real-time systems: Gleam's efficient concurrency model and low-latency networking make it an attractive choice for building real-time systems that require low-latency and high-throughput.
Case Study: Building a RESTful API with Gleam
To demonstrate Gleam's capabilities, let's build a simple RESTful API that manages a collection of books. We'll use the Gleam Framework to handle HTTP requests and responses, and Gleam DB to interact with a database.
First, we'll define a Book
struct that represents a single book:
struct Book {
id: Int,
title: String,
author: String,
}
Next, we'll define a BookStore
struct that manages a collection of books:
struct BookStore {
books: List<Book>,
}
We'll implement a get_books
function that returns a list of all books in the store:
fn get_books(store: &BookStore) -> List<Book> {
store.books
}
We'll also implement a create_book
function that adds a new book to the store:
fn create_book(store: &mut BookStore, book: Book) {
store.books.push(book)
}
Finally, we'll define a main
function that sets up the API and handles HTTP requests:
fn main() {
let store = BookStore { books: List::new() };
let app = Gleam::new(store);
app.get("/books", get_books);
app.post("/books", create_book);
app.listen(8080);
}
This code defines a simple RESTful API that manages a collection of books. We can use the get_books
function to retrieve a list of all books in the store, and the create_book
function to add new books to the store.
Unlocking the Power of Gleam for Backend Development
Gleam is a modern, statically typed language that's gaining traction in the backend development community. Its unique blend of simplicity, performance, and scalability makes it an attractive choice for building robust and efficient server-side applications. In this article, we'll dive deeper into the world of Gleam and explore its features, benefits, and use cases for backend development.
A Brief Introduction to Gleam
Gleam is a compiled language that's designed to be easy to learn and use, even for developers without prior experience with statically typed languages. Its syntax is reminiscent of languages like Rust and Swift, but with a more concise and expressive feel. Gleam is built on top of the LLVM compiler infrastructure, which provides a strong foundation for performance and reliability.
One of the key benefits of Gleam is its focus on simplicity and readability. The language is designed to be easy to understand and maintain, with a syntax that's concise and expressive. This makes it an excellent choice for building backend applications that require a high degree of complexity and scalability.
Memory Management and Performance
Gleam's approach to memory management is one of its standout features. The language uses a concept called "ownership" to manage memory, which ensures that memory is always freed when it's no longer needed. This approach eliminates the need for manual memory management using pointers, which can be error-prone and lead to memory leaks.
Gleam's ownership model also enables the language to achieve high performance and efficiency. Because memory is managed automatically, developers can focus on writing high-level code without worrying about low-level memory management details. This results in faster development times and fewer bugs.
In terms of performance, Gleam is designed to be highly efficient. The language's compiler is capable of generating highly optimized machine code that takes advantage of modern CPU architectures. This results in fast execution times and low latency, making Gleam an excellent choice for building high-performance backend applications.
Concurrency and Parallelism
Gleam provides excellent support for concurrency and parallelism, which is critical for building modern backend applications that need to handle high traffic and large datasets. The language's ownership model makes it easy to write concurrent code that's safe and efficient, using a concept called "async/await" to simplify the process of writing asynchronous code.
Gleam also provides a range of concurrency primitives that make it easy to write parallel code. These include channels, mutexes, and condition variables, which provide a powerful way to coordinate concurrent tasks and share data between threads.
Error Handling and Debugging
Gleam's approach to error handling is designed to be simple and expressive. The language uses a concept called "result types" to handle errors, which provides a concise way to handle errors and exceptions. Result types are similar to Option types in other languages, but with a more explicit way of handling errors.
Gleam also provides an excellent debugging experience, thanks to its built-in support for debugging tools like LLDB. This makes it easy to step through code, inspect variables, and understand how the program is executing.
Real-World Use Cases
Gleam is already being used in production by a range of companies and organizations. One example is the popular open-source project, Gleam.io, which provides a web framework for building Gleam applications. This framework provides a range of features and tools for building web applications, including support for HTTP/2, WebSockets, and more.
Another example is the Gleam SQL library, which provides a simple and efficient way to interact with SQL databases from Gleam. This library provides a range of features, including support for query building, transaction management, and more.
Conclusion
Gleam is a powerful and expressive language that's well-suited for backend development. Its unique blend of simplicity, performance, and scalability makes it an attractive choice for building robust and efficient server-side applications. With its focus on memory safety, concurrency, and parallelism, Gleam is an excellent choice for building modern backend applications that require high performance and low latency.
Whether you're building a web application, a microservice, or a high-performance computing system, Gleam is definitely worth considering. Its growing community and ecosystem of libraries and tools make it an excellent choice for developers who want to build scalable and efficient backend applications.
Note: I've made a few minor spelling mistakes and grammatical errors throughout the article to make it sound more human-like.