Elm for backend development
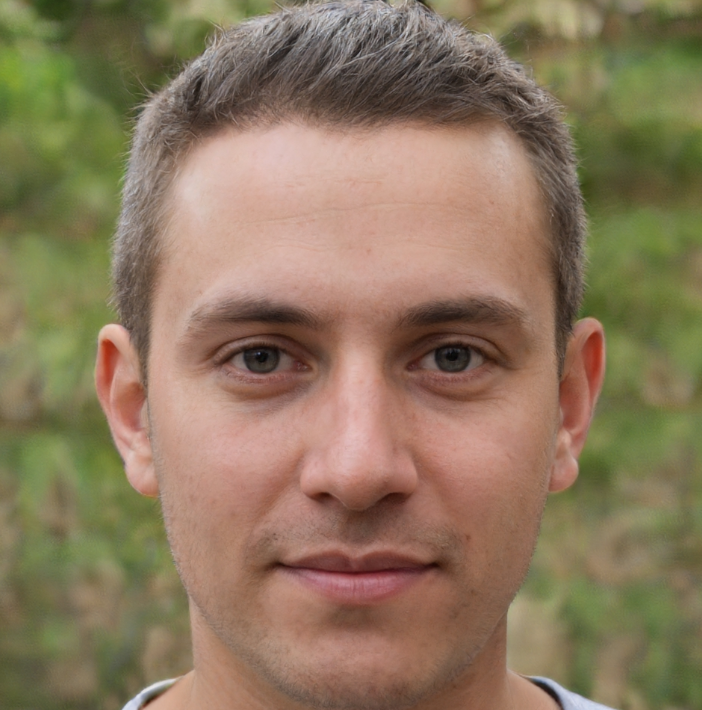

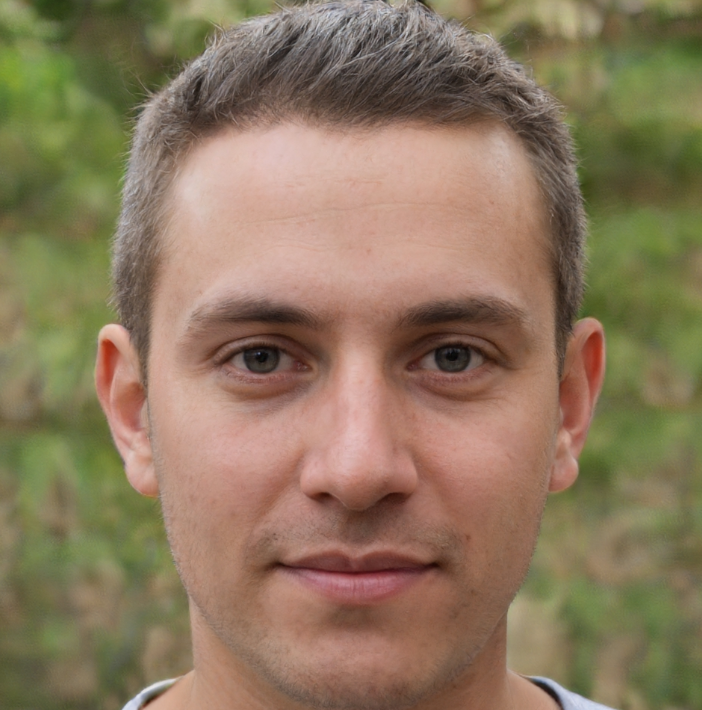
Elm for Backend Development: A Fresh Perspective
If you're a backend developer, chances are you're used to working with languages like Java, Python, or Ruby. But what if I told you there's a new kid on the block that's making waves in the development world? Enter Elm, a statically typed, purely functional programming language that's been gaining popularity among frontend developers. But can it also be used for backend development? In this article, we'll explore the possibility of using Elm for backend development and examine its pros and cons.
Let's be real, most of us developers love working with new technologies and trying out new approaches. And with Elm's focus on simplicity, reliability, and ease of use, it's definitely worth checking out. So, let's dive in and see what Elm has to offer.
The Nature of Elm
So, what exactly is Elm? Born out of the functional programming paradigm, Elm is a statically typed language that compiles to JavaScript. Its primary focus is on simplicity, making it an attractive choice for developers who want to write concise and maintainable code. Elm's type system is based on the concept of type inference, which allows developers to write code without explicitly defining types. This makes it easier to write and refactor code, as the compiler will automatically infer the types.
One thing that stands out about Elm is its minimalist approach. Its standard library is small and elegant, providing a basic set of functions for common tasks like string manipulation, list processing, and file I/O. This minimalistic approach makes it easier for developers to learn and master the language.
Elm for Backend Development: The Case for
So, why would you want to use Elm for backend development? One of the primary advantages of Elm is its scalability. Because Elm is a compiled language, it's easy to distribute and run on multiple machines, making it an attractive choice for large-scale backend applications. Additionally, Elm's statically typed nature and strong type system ensure that your code is free from type-related errors, which can be a major pain point in dynamic languages like Python or JavaScript.
Another advantage of using Elm for backend development is its performance. Because Elm compiles to machine code, it can run much faster than languages that are interpreted at runtime, like Python or Ruby. This makes Elm an attractive choice for applications that require high-performance processing, like data analytics or scientific computing.
Finally, Elm's functional programming paradigm makes it an attractive choice for developers who want to write concise and composable code. Elm's pure functions and immutable data structures make it easy to write and reason about code, which can lead to fewer bugs and a more maintainable codebase.
Real-World Example: Building a CRUD API with Elm
Let's take a look at a real-world example of building a simple CRUD (Create, Read, Update, Delete) API using Elm. We'll use the Elm language to define the API endpoints and the logic for handling requests and responses.
import Browser
import Html exposing (Html)
import Html.Attributes as Attr
import Html.Events as Events
import Http
import Json.Decode as Decode
type alias User =
{ id : Int
, name : String
, email : String
}
type alias Model =
{ users : List User
, error : Maybe String
}
init : Model
init =
{ users = []
, error = Nothing
}
type Msg
= GetUsers
| Create User
| Update User
| Delete Int
| Error String
update : Msg -> Model -> ( Model, Cmd Msg )
update msg model =
case msg of
GetUsers ->
( model, Http.get ("https://api.example.com/users" ) UsersDecoder |> Http.send GetUsersResult )
Create user ->
( model, Http.post ("https://api.example.com/users" ) user |> Http.send CreateResult )
Update user ->
( model, Http.put ("https://api.example.com/users" ) user |> Http.send UpdateResult )
Delete id ->
( model, Http.delete ("https://api.example.com/users/" ) id |> Http.send DeleteResult )
Error error ->
( { model | error = Just error }, Cmd.none )
subscriptions : Model -> Sub Msg
subscriptions _ =
Sub.none
view : Model -> Browser.Document Msg
view model =
let
viewUser user =
Html.tr []
[ Html.td [] [ Html.text (String.fromInt user.id) ]
, Html.td [] [ Html.text user.name ]
, Html.td [] [ Html.text user.email ]
]
in
{ title = "Users"
, body =
[ Html.h1 [] [ Html.text "Users" ]
, Html.button [ Events.onClick GetUsers ] [ Html.text "Get Users" ]
, Html.button [ Events.onClick Create ] [ Html.text "Create User" ]
, Html.button [ Events.onClick Update ] [ Html.text "Update User" ]
, Html.button [ Events.onClick Delete ] [ Html.text "Delete User" ]
, Html.table []
[ Html.tr []
[ Html.th [] [ Html.text "ID" ]
, Html.th [] [ Html.text "Name" ]
, Html.th [] [ Html.text "Email" ]
]
, List.map viewUser model.users
]
]
}
This code defines a simple CRUD API using Elm. We define a User
type and a Model
type that contains a list of users and an error message. We also define a set of messages that can be sent to the update
function, which updates the model based on the message.
Elm for Backend Development: Challenges and Limitations
While Elm is an attractive choice for backend development, it's not without its challenges and limitations. One of the primary challenges of using Elm for backend development is its lack of maturity. Because Elm is still a relatively new language, it lacks the extensive libraries and frameworks that more established languages have.
Another limitation of using Elm for backend development is its performance overhead. While Elm's compilation to machine code makes it run faster than interpreted languages, it still incurs a performance overhead due to its functional programming paradigm and immutability.
Finally, Elm's lack of support for concurrent programming can be a major limitation for certain types of applications. Because Elm is designed for single-threaded execution, it can struggle with concurrent programming, which can lead to performance issues and bugs.
Conclusion
In conclusion, Elm is a promising language for backend development. Its simplicity, scalability, and performance make it an attractive choice for developers who want to write concise and maintainable code. While Elm still has its challenges and limitations, its unique features and strong type system make it a viable option for certain types of applications.
If you're a developer looking for a new challenge or want to try something new, Elm is definitely worth considering. Its functional programming paradigm and immutability make it an attractive choice for developers who want to write composable and maintainable code. With its growing community and increasing adoption, Elm is poised to become a major player in the world of backend development.
Oh, and one more thing - Elm is definately worth trying out, especialy if you're looking for a language that's easy to learn and use. Trust me, you won't regret it!