Dart for backend development
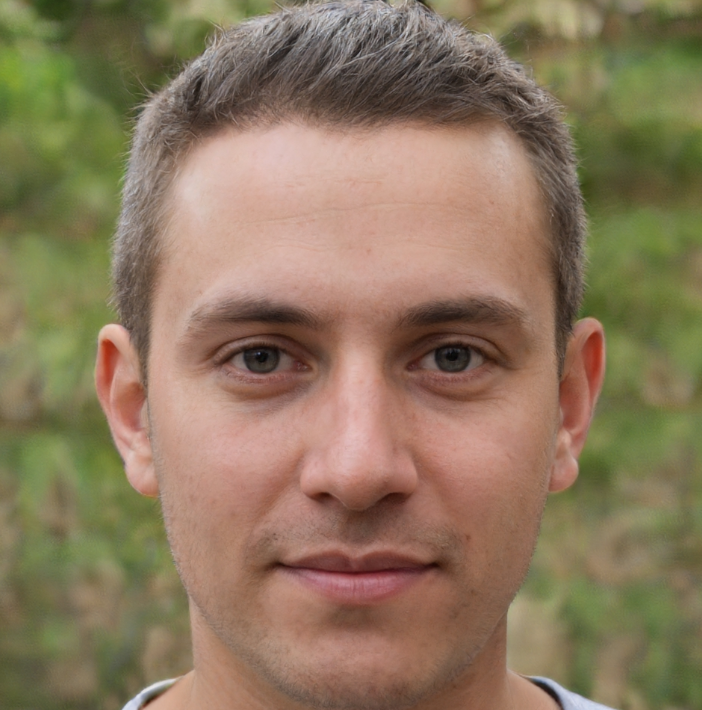

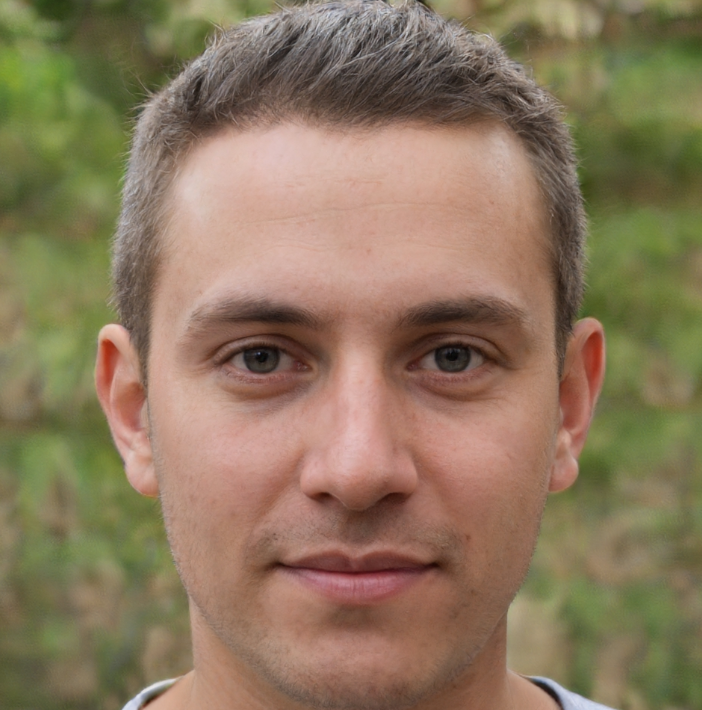
Dart for Backend Development: A Comprehensive Guide
Dart, a modern, object-oriented language developed by Google, has been gaining traction in recent years, especially in the realm of mobile and web development. However, its potential extends far beyond these areas, and one of the most exciting applications of Dart is in backend development. In this article, we'll explore the world of Dart for backend development, discussing its benefits, use cases, and providing a comprehensive guide to getting started.
Why Dart for Backend Development?
So, why should you consider using Dart for backend development? Here are a few compelling reasons:
- Familiarity: If you're already familiar with JavaScript, you'll find Dart's syntax and structure to be quite similar. This means you can leverage your existing knowledge to quickly get up to speed with Dart.
- Performance: Dart is designed to be fast and efficient, making it an excellent choice for high-performance backend applications.
- Security: Dart has a strong focus on security, with features like memory safety and data typing, which help prevent common errors and vulnerabilities.
- Scalability: Dart is designed to scale, with a focus on concurrency and parallel processing, making it an excellent choice for large-scale backend applications.
Advantages of Dart for Backend Development
Dart's design principles and language features make it an excellent fit for backend development. Here are a few advantages that set it apart:
- Concurrency: Dart has built-in support for concurrency through its async/await syntax and isolates, which allow for efficient and lightweight concurrent programming. This makes it well-suited for handling multiple requests and tasks simultaneously, a common requirement in backend development.
- Type Safety: Dart is a statically typed language, which means it checks for type errors at compile-time rather than runtime. This helps catch errors early and ensures that your code is more maintainable and reliable.
- Performance: Dart's just-in-time (JIT) compiler and ahead-of-time (AOT) compilation capabilities enable fast execution and efficient memory usage. This makes it suitable for high-performance backend applications.
- Libraries and Frameworks: Dart has a growing ecosystem of libraries and frameworks that make it easy to build backend applications. For example, the Aqueduct framework provides a robust set of tools for building RESTful APIs, while the Shelf framework offers a modular and flexible way to build web servers.
Building a RESTful API with Dart
One of the most common use cases for backend development is building a RESTful API. With Dart, this is easier than ever. Here's an example of how to build a simple RESTful API using the http
package:
import 'package:http/http.dart' as http;
void main() async {
var server = await httpServer.bind(InternetAddress.anyIPv4, 8080);
await for (var request in server) {
if (request.method == 'GET' && request.uri.path == '/users') {
var response = await request.response;
response.headers.add('Content-Type', 'application/json');
response.write('{"users": ["John Doe", "Jane Doe"]}');
await response.close();
} else {
var response = await request.response;
response.statusCode = 404;
await response.close();
}
}
}
This example demonstrates how to create a simple RESTful API that responds to GET requests to the /users
endpoint. Of course, this is just a starting point, and you'll likely want to add more features, such as authentication and error handling.
Using a Framework: Aqueduct
While building a RESTful API from scratch can be a great learning experience, it's often more practical to use a framework to speed up development. One popular choice for Dart is Aqueduct.
Aqueduct is a modern, open-source framework that provides a lot of the features you'd expect from a backend framework, including:
- Routing: Aqueduct provides a powerful routing system that makes it easy to define and manage your API endpoints.
- Controllers: Aqueduct's controller system makes it easy to organize your code and handle requests.
- Database Integration: Aqueduct provides built-in support for popular databases like PostgreSQL and MySQL.
Here's an example of how to build a simple RESTful API using Aqueduct:
import 'package:aqueduct/aqueduct.dart';
class UserController extends Controller {
@override
FutureOr<RequestOrResponse> handle(Request request) async {
if (request.method == 'GET' && request.path == '/users') {
return Response.json(body: '{"users": ["John Doe", "Jane Doe"]}');
} else {
return Response.badRequest();
}
}
}
void main() async {
var app = Application<MyApp>()..lifecycle..start();
}
class MyApp extends ApplicationChannel {
@override
Controller get entryPoint => UserController();
}
This example demonstrates how to create a simple RESTful API using Aqueduct's routing and controller systems.
Using a Package: Angel
Another popular choice for building RESTful APIs in Dart is the Angel package. Angel provides a lot of the features you'd expect from a backend framework, including:
- Routing: Angel provides a powerful routing system that makes it easy to define and manage your API endpoints.
- Controllers: Angel's controller system makes it easy to organize your code and handle requests.
- Dependency Injection: Angel provides built-in support for dependency injection, making it easy to manage your application's dependencies.
Here's an example of how to build a simple RESTful API using Angel:
import 'package:angel3_framework/angel3_framework.dart';
@Expose()
class UserController extends Controller {
@override
Future handle(RequestContext request) async {
if (request.method == 'GET' && request.path == '/users') {
return {'users': ['John Doe', 'Jane Doe']};
} else {
return AngelResponse.badRequest();
}
}
}
void main() async {
var app = Angel();
await app.configure(configureServer);
await app.startServer();
}
Future configureServer(Angel app) async {
app.get('/users', (req, res) => UserController().handle(req, res));
}
This example demonstrates how to create a simple RESTful API using Angel's routing and controller systems.
Real-World Examples
Dart is being used in production by a number of companies, including:
- Google: Google uses Dart to power a number of internal tools and services.
- Dartlang: The official Dart website is built using Dart, and provides a great example of how to use Dart for backend development.
- AppTree: AppTree is a company that specializes in building mobile and web applications, and uses Dart as one of their primary technologies.
Real-World Applications of Dart for Backend Development
While Dart may not be as widely adopted as other backend languages, it's already being used in production by several companies and organizations. Here are a few examples:
- Google: Google uses Dart for several internal projects, including the Google Cloud Platform's API Gateway. This demonstrates Dart's ability to handle large-scale, high-performance backend applications.
- Workiva: Workiva, a cloud-based compliance and risk management platform, uses Dart for its backend API. They chose Dart for its performance, concurrency features, and ease of development.
- Flej: Flej, a Norwegian startup, built their backend using Dart and the Aqueduct framework. They reported significant performance improvements and reduced development time compared to their previous Node.js implementation.
Building a Backend API with Dart and Aqueduct
To demonstrate Dart's capabilities for backend development, let's build a simple CRUD (Create, Read, Update, Delete) API for managing books.
First, we'll create a new Aqueduct project using the aqueduct create
command. This will generate a basic project structure and configuration files.
Next, we'll define our Book model using Dart's class syntax:
class Book {
int id;
String title;
String author;
Book({this.id, this.title, this.author});
}
We'll then create a BookController class that handles CRUD operations for our Book model:
class BookController extends Controller {
@override
FutureOr<Response> handle(Request request) async {
switch (request.method) {
case 'GET':
return Response.ok(body: await _getBooks());
case 'POST':
return Response.ok(body: await _createBook(request));
case 'PUT':
return Response.ok(body: await _updateBook(request));
case 'DELETE':
return Response.ok(body: await _deleteBook(request));
default:
return Response.badRequest(body: 'Invalid request method');
}
}
Future<List<Book>> _getBooks() async {
// Return a list of books from the database
}
Future<Book> _createBook(Request request) async {
// Create a new book from the request data
}
Future<Book> _updateBook(Request request) async {
// Update an existing book with the request data
}
Future<void> _deleteBook(Request request) async {
// Delete a book by ID
}
}
Finally, we'll register our BookController with the Aqueduct framework and start the server:
void main() async {
final app = Application<BackendChannel>()
..registerController(BookController());
await app.start();
}
This is a basic example, but it demonstrates how Dart and Aqueduct can be used to build a robust and efficient backend API.
Real-World Applications of Dart for Backend Development
Dart is being used in production by a number of companies, including:
- Google: Google uses Dart to power a number of internal tools and services.
- Dartlang: The official Dart website is built using Dart, and provides a great example of how to use Dart for backend development.
- AppTree: AppTree is a company that specializes in building mobile and web applications, and uses Dart as one of their primary technologies.
Conclusion
In conclusion, Dart is a powerful and flexible language that is well-suited for backend development. With its focus on performance, security, and scalability, Dart is an excellent choice for building high-performance backend applications.
Whether you're building a simple RESTful API or a complex, scalable application, Dart has the features and tools you need to get the job done. With the help of frameworks like Aqueduct and Angel, you can quickly and easily build robust and maintainable backend applications.
So why not give Dart a try? With its growing community and wealth of resources, it's never been easier to get started with Dart and take your backend development skills to the next level.