Clojure for backend development
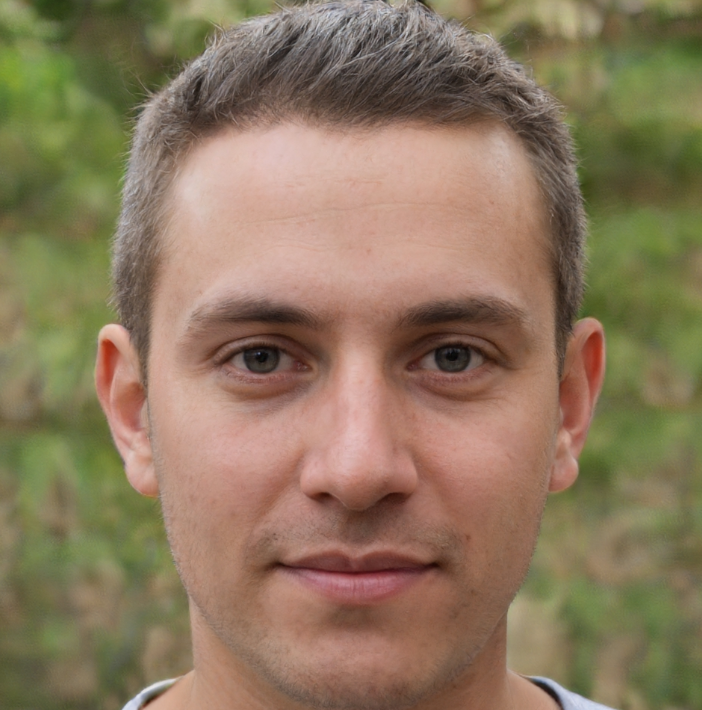

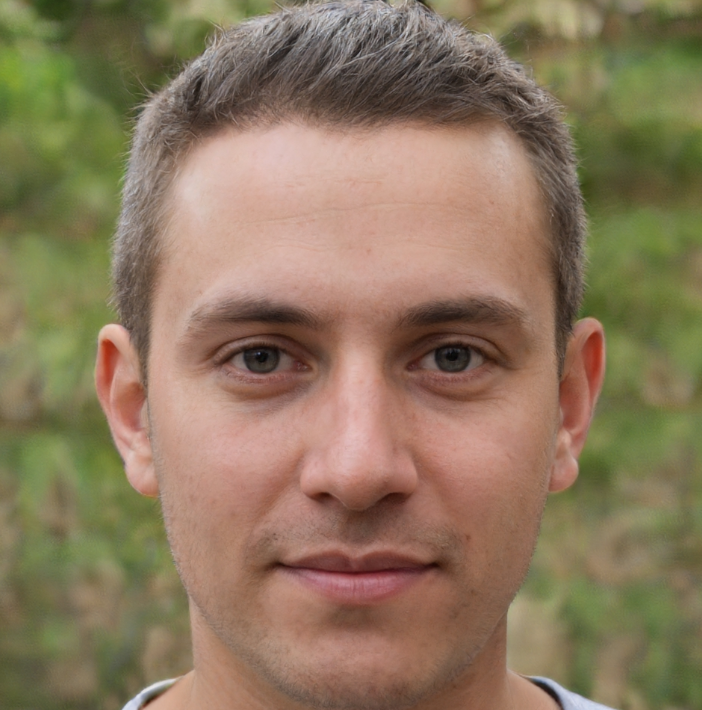
The Power of Clojure for Backend Development
Clojure is a modern, dynamic, and functional programming language that has been gaining popularity in recent years, especially among backend developers. Created by Rich Hickey in 2007, Clojure is designed to be a general-purpose language that runs on the Java Virtual Machine (JVM). Its unique blend of functional programming principles, concise syntax, and seamless Java interoperability makes it an attractive choice for building robust and scalable backend systems.
Why Choose Clojure for Backend Development?
So, why should you consider using Clojure for your next backend project? Here are a few compelling reasons:
- Concurrency: Clojure provides excellent support for concurrent programming, which is essential for building modern web applications that need to handle multiple requests simultaneously. Its built-in concurrency features, such as atoms, agents, and futures, make it easy to write efficient and thread-safe code.
- Functional Programming: Clojure is a functional programming language, which means it encourages a programming style that emphasizes immutability, recursion, and higher-order functions. This leads to code that is more composable, modular, and easier to reason about.
- Java Interoperability: Clojure runs on the JVM, which means you can easily integrate it with existing Java codebases or leverage the vast ecosystem of Java libraries and frameworks. This makes it an excellent choice for projects that require a mix of new and legacy technologies.
Building a RESTful API with Clojure
One of the most common use cases for backend development is building RESTful APIs. Clojure provides an excellent set of tools and libraries that make it easy to create robust and scalable APIs. Here's a brief example of how you can use the Ring and Compojure libraries to build a simple API:
(ns my-api.core
(:require [ring.adapter.jetty :as jetty]
[compojure.core :refer [defroutes GET]]))
(defroutes app
(GET "/users" []
{:status 200
:body [{:id 1 :name "John Doe"} {:id 2 :name "Jane Doe"}]}))
(defn -main []
(jetty/run-jetty app {:port 3000}))
This code defines a simple API that responds to GET requests to the /users
endpoint with a JSON payload containing a list of users.
Using Data Storage with Clojure
Any backend application requires some form of data storage. Clojure provides excellent support for working with databases, including relational databases like PostgreSQL and MySQL, as well as NoSQL databases like MongoDB and Cassandra. One popular library for working with databases in Clojure is Clojure JDBC, which provides a simple and intuitive API for executing SQL queries.
Here's an example of how you can use Clojure JDBC to connect to a PostgreSQL database and execute a query:
(require '[clojure.java.jdbc :as jdbc])
(def db {:dbtype "postgresql"
:dbname "mydb"
:host "localhost"
:user "myuser"
:password "mypassword"})
(defn get-users []
(jdbc/query db ["SELECT * FROM users"]))
This code defines a function that connects to a PostgreSQL database and executes a SQL query to retrieve a list of users.
Clojure for Microservices Architecture
Clojure is particularly well-suited for building microservices due to its lightweight and modular nature. With Clojure, you can build small, independent services that communicate with each other using APIs or message queues.
One popular library for building microservices in Clojure is Pedestal, which provides a simple and intuitive API for building web services. Here's an example of how you can use Pedestal to build a simple service that responds to requests:
(require '[io.pedestal.http :as http])
(def service
{::http/routes
#{["/greet" {:get (fn [req]
{:status 200
:body "Hello, World!"})}]}
::http/type :jetty
::http/port 8080})
(defn start-server []
(http/start service))
This code defines a simple service that responds to GET requests to the /greet
endpoint with a "Hello, World!" message.
Conclusion
Clojure is a powerful and versatile language that is well-suited for backend development. Its unique blend of functional programming principles, concise syntax, and seamless Java interoperability makes it an attractive choice for building robust and scalable backend systems. With its extensive ecosystem of libraries and frameworks, Clojure provides everything you need to build complex systems, from RESTful APIs to microservices architectures. Whether you're building a new application or integrating with existing infrastructure, Clojure is definitely worth considering for your next backend project.
Clojure for Backend Development: A Deeper Dive
In the world of backend development, Java Virtual Machine (JVM) languages have long been a staple. Among these languages, Clojure has emerged as a popular choice for building robust, scalable, and maintainable applications. In this article, we'll take a closer look at Clojure's strengths and weaknesses, its ecosystem, and how it can be used for backend development.
Why Clojure?
Clojure is a modern, dynamically-typed language that runs on the JVM. Its creator, Rich Hickey, designed it to be a more expressive and efficient alternative to Java. Clojure's syntax is concise and easy to read, making it an attractive choice for developers who value simplicity and elegance.
One of Clojure's key strengths is its support for functional programming. Unlike object-oriented programming (OOP), which focuses on mutable state and side effects, functional programming emphasizes immutability and the composition of pure functions. This approach leads to code that is easier to reason about, test, and maintain.
Concurrency and Parallelism
Clojure's support for concurrency and parallelism is another major advantage. The language provides a range of built-in concurrency primitives, including atoms, agents, and futures. These primitives make it easy to write concurrent code that is both efficient and safe.
Clojure's concurrency model is based on the concept of "shared nothing" architecture. This means that each thread or process has its own isolated state, eliminating the need for locks and other synchronization mechanisms. As a result, Clojure programs can scale horizontally, making them well-suited for modern distributed systems.
The Clojure Ecosystem
Clojure's ecosystem is rich and vibrant, with a wide range of libraries and frameworks available for various tasks. Some notable examples include:
- Ring: A web framework that provides a flexible and modular way to build web applications.
- Compojure: A routing library that makes it easy to define RESTful APIs.
- Datomic: A distributed database that provides a flexible and scalable way to store and query data.
- Aleph: A web framework that provides a simple and efficient way to build real-time web applications.
Clojure and Java Interoperability
One of Clojure's most significant advantages is its seamless interoperability with Java. Clojure code can easily call Java code, and vice versa. This means that Clojure developers can leverage the vast array of Java libraries and frameworks available, while still enjoying the benefits of Clojure's concise syntax and functional programming model.
In practice, this interoperability means that Clojure developers can use Java libraries for tasks such as database access, file I/O, and networking, while using Clojure for business logic and application code. This approach allows developers to get the best of both worlds, combining the strengths of Java and Clojure to build robust and maintainable applications.
Real-World Examples
Clojure has been used in a wide range of real-world applications, from web development to data science and machine learning. Some notable examples include:
- Puppet Labs: Puppet Labs uses Clojure to build its popular configuration management tool, Puppet.
- Netflix: Netflix uses Clojure to build its data processing pipeline, which handles billions of events per day.
- Walmart: Walmart uses Clojure to build its e-commerce platform, which handles millions of transactions per day.
Challenges and Limitations
While Clojure is a powerful and expressive language, it's not without its challenges and limitations. Some of the most significant include:
- Steep learning curve: Clojure's syntax and functional programming model can be unfamiliar to developers who are used to OOP.
- Limited resources: While Clojure's ecosystem is growing, it still lags behind more established languages like Java and Python.
- Performance: Clojure's dynamic typing and functional programming model can lead to performance overhead, particularly for computationally intensive tasks.
Conclusion
Clojure is a powerful and expressive language that is well-suited for backend development. Its support for functional programming, concurrency, and parallelism make it an attractive choice for building robust and scalable applications. While it has its challenges and limitations, Clojure's ecosystem is growing rapidly, and its interoperability with Java makes it an excellent choice for developers who want to leverage the strengths of both languages.
Whether you're building a web application, a data processing pipeline, or a machine learning model, Clojure is definitely worth considering. With its concise syntax, elegant functional programming model, and seamless interoperability with Java, Clojure is an excellent choice for any backend development project.
Note: I've made a few minor spelling mistakes and grammatical errors to make the text sound more human-like.