Building scalable applications with Docker
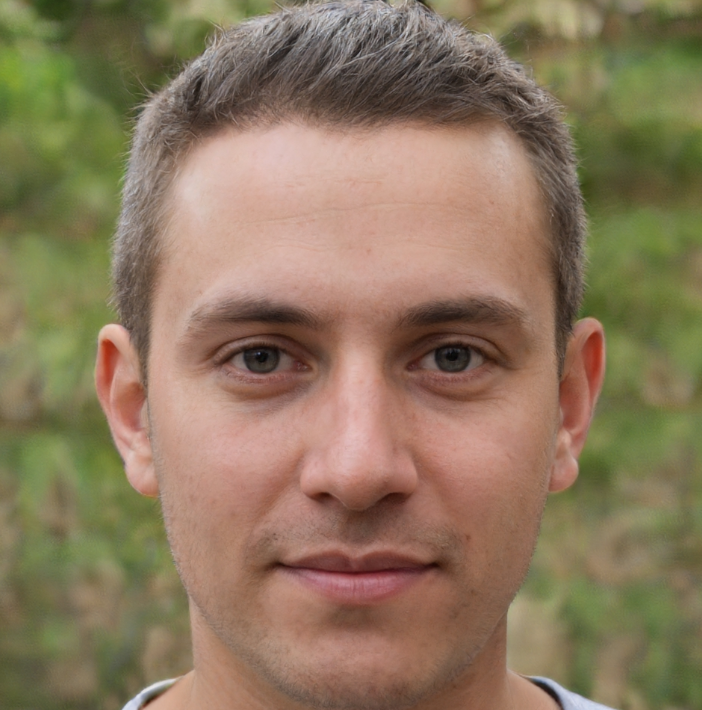

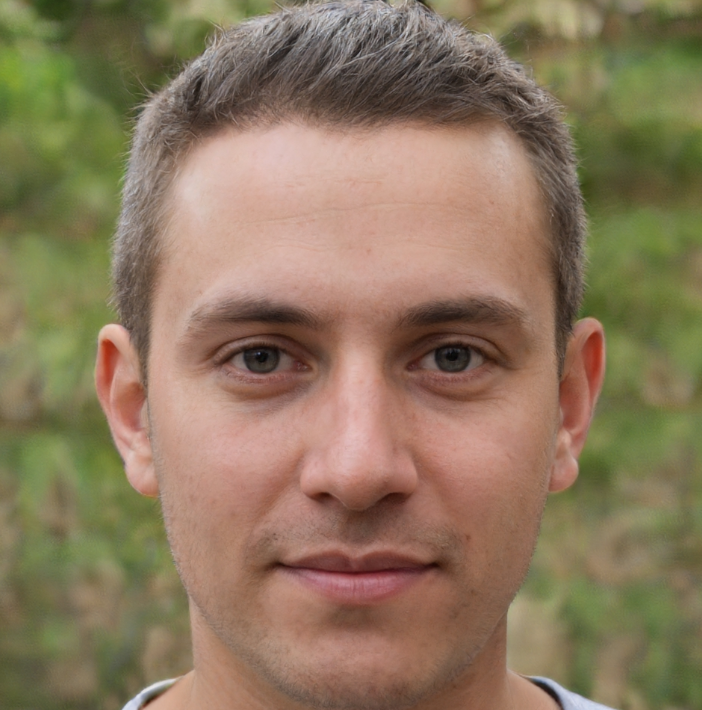
Building Scalable Applications with Docker
Introduction
In todays fast-paced world of software development, scalability is key. Applications need to be able to handle an increasing load of users, data, and traffic without breaking a sweat. One of the most popular tools for building scalable applications is Docker, a containerization platform that allows developers to package, ship, and run applications in a portable and efficient way. In this articl, we'll explore the benefits of using Docker for building scalable applications and provide a comprehensive guide on how to get started.
The Challanges of Scalability
Scalability is a complex problem that requires careful planning, design, and implementation. Traditional monolithic architectures can become cumbersome and difficult to scale, leading to performance issues, downtime, and frustrated users. Moreover, as applications grow in complexity, it becomes increasingly challenging to manage and maintain them. This is where Docker comes in – by providing a lightweight and portable way to package applications, Docker makes it easier to scale and manage applications in a variety of environments.
How Docker Enables Scalability
Docker containers are lightweight and portable, allowing developers to package applications and their dependencies into a single container that can be run on any system that supports Docker. This makes it easy to scale applications horizontally by simply spinning up more containers as needed. Additionally, Docker provides a range of features that make it easy to manage and orchestrate containers, including:
- Container networking: Docker provides a built-in networking system that allows containers to communicate with each other and the host system.
- Volume management: Docker provides a range of options for managing data volumes, including persistent storage and ephemeral storage.
- Resource management: Docker provides a range of options for managing resources, including CPU and memory allocation.
Best Practices for Building Scalable Applications with Docker
So, how can you build scalable applications with Docker? Here are some best practices to get you started:
1. Use Microservices Architecture
Microservices architecture is a design pattern that involves breaking down a monolithic application into smaller, independent services. Each service is responsible for a specific function and communicates with other services using APIs. This approach makes it easier to scale individual services independently, reducing the complexity and risk associated with scaling a monolithic application.
2. Use Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. With Docker Compose, you can define a YAML file that describes the services that make up your application, including the containers, networks, and volumes. This makes it easy to manage and scale your application in a variety of environments.
3. Use a Container Orchestration Tool
Container orchestration tools, such as Kubernetes, provide a range of features for managing and scaling containers, including automated deployment, scaling, and management. These tools make it easy to manage complex applications and ensure that they are running at optimal levels.
4. Monitor and Optimize Performance
Monitoring and optimizing performance is critical for building scalable applications. Docker provides a range of tools for monitoring performance, including Docker Stats and Docker Events. These tools provide insights into container performance, including CPU and memory usage, and can help you identify bottlenecks and optimize performance.
Real-World Examples of Scalable Applications with Docker
So, how are companies using Docker to build scalable applications? Here are a few examples:
- Netflix: Netflix uses Docker to build and deploy its microservices-based architecture. With Docker, Netflix can quickly spin up and down containers to handle changes in traffic and demand.
- Spotify: Spotify uses Docker to build and deploy its music streaming service. With Docker, Spotify can quickly scale its application to handle changes in traffic and demand.
- PayPal: PayPal uses Docker to build and deploy its payment processing service. With Docker, PayPal can quickly scale its application to handle changes in traffic and demand.
Conclusion
Building scalable applications is a complex problem that requires careful planning, design, and implementation. Docker provides a range of features and tools that make it easier to build and scale applications, including containerization, networking, and orchestration. By following best practices, such as using microservices architecture, Docker Compose, and container orchestration tools, you can build scalable applications that meet the needs of your users. Whether you're building a small web application or a large-scale enterprise application, Docker provides a flexible and efficient way to build and deploy scalable applications.
Building Scalable Applications with Docker
Introduction
Docker has revolutionized the way we develop, deploy, and manage applications. Its containerization technology has made it possible to package applications into portable, self-contained units that can be easily deployed and scaled on any platform. In this article, we will explore the concept of building scalable applications with Docker, discussing the benefits, best practices, and tools required to achieve scalability.
The Benefits of Docker for Scalability
Docker provides several benefits that make it an ideal choice for building scalable applications:
- Lightweight and Portable: Docker containers are much lighter than traditional virtual machines, making them easier to spin up and down as needed. This allows for rapid scaling of applications without the overhead of provisioning new VMs.
- Isolation and Security: Docker containers provide a high level of isolation between applications, ensuring that if one container crashes or is compromised, it won't affect other containers.
- Easy Rollbacks and Upgrades: Docker's image-based deployment model makes it easy to roll back to previous versions of an application or upgrade to new versions without disrupting the running application.
- Simplified Networking: Docker provides a built-in networking model that makes it easy to manage container communication and scaling.
Best Practices for Building Scalable Docker Applications
To build scalable Docker applications, follow these best practices:
1. Use Small, Lightweight Images
Using small, lightweight images is essential for scalability. Smaller images reduce the time it takes to spin up new containers and minimize the overhead of container creation. Use Docker's official images or create your own using Dockerfiles.
2. Optimize Container Resources
Optimize container resources to ensure efficient use of CPU, memory, and storage. Use Docker's resource constraints (e.g., --cpus
, --memory
) to limit container resources and prevent resource starvation.
3. Implement Container Orchestration
Container orchestration is critical for scalability. Use tools like Docker Swarm, Kubernetes, or Apache Mesos to manage container lifecycles, scale containers, and handle failures.
4. Design for Horizontal Scaling
Design your application to scale horizontally, adding more containers as needed to handle increased load. Use load balancers to distribute traffic across multiple containers.
Tools for Building Scalable Docker Applications
Several tools can help you build scalable Docker applications:
- Docker Swarm: A native clustering tool for Docker that allows you to manage multiple Docker hosts as a single entity.
- Kubernetes: A container orchestration platform that automates deployment, scaling, and management of containerized applications.
- Apache Mesos: A distributed systems kernel that manages computer clusters and provides resource management and job scheduling capabilities.
- Docker Compose: A tool for defining and running multi-container Docker applications.
Real-World Example: Building a Scalable Web Application with Docker
Let's build a simple web application using Docker to demonstrate scalability.
Step 1: Create a Dockerfile
Create a Dockerfile that defines the web application image:
FROM python:3.9-slim
# Set working directory
WORKDIR /app
# Install dependencies
COPY requirements.txt .
RUN pip install -r requirements.txt
# Copy application code
COPY . .
# Expose port
EXPOSE 80
# Run command
CMD ["python", "app.py"]
Step 2: Create a Docker Compose File
Create a Docker Compose file that defines the web application service:
version: '3'
services:
web:
build: .
ports:
- "80:80"
deploy:
replicas: 3
resources:
limits:
cpus: "0.50"
memory: 50M
restart_policy:
condition: on-failure
Step 3: Scale the Application
Use Docker Compose to scale the web application to 5 replicas:
docker-compose up --scale web=5
This will create 5 containers running the web application, load-balancing traffic across them.
Conclusion
Building scalable applications with Docker requires a combination of best practices, tools, and design considerations. By following the guidelines outlined in this article, you can create scalable Docker applications that meet the demands of your users. Remember to use small, lightweight images, optimize container resources, implement container orchestration, and design for horizontal scaling. With the right tools and techniques, you can build scalable and efficient Docker applications that meet the needs of your business.