Building a scalable backend with Spring Boot
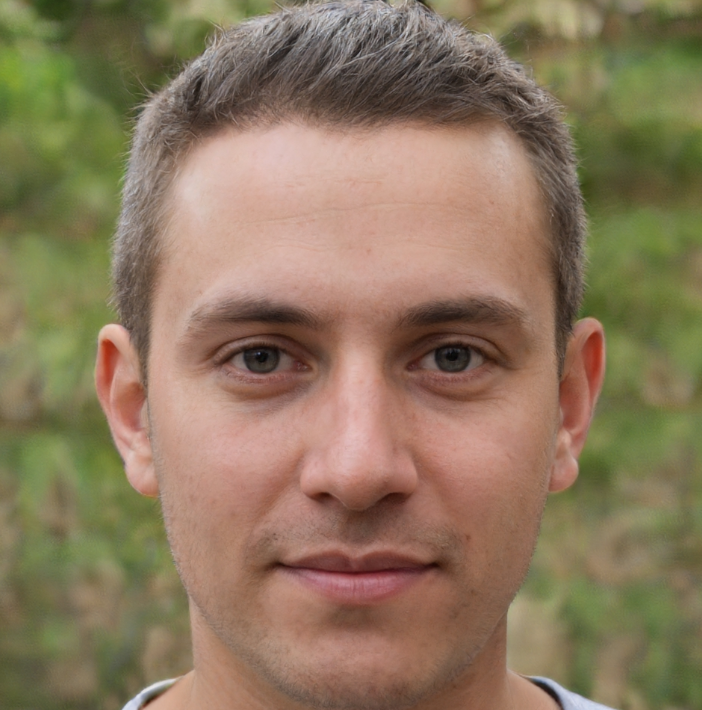

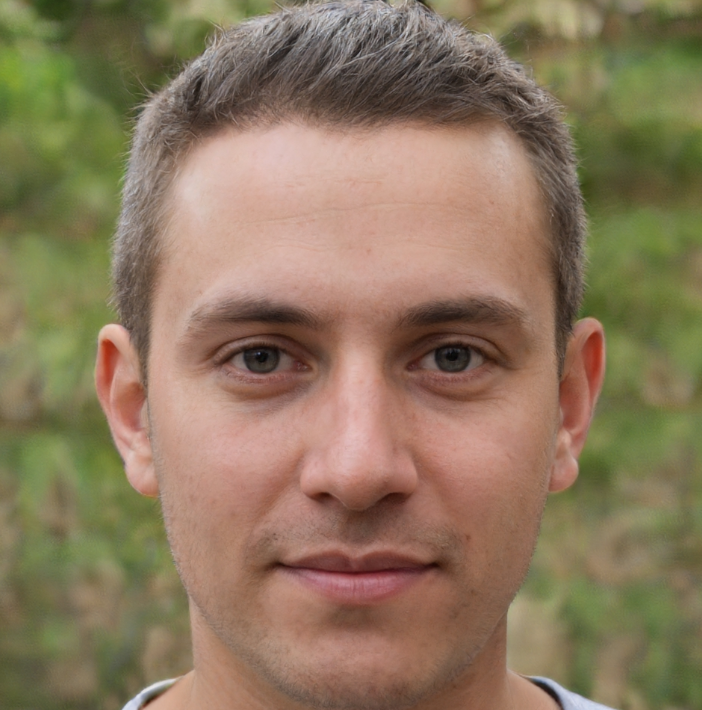
Building a Scalable Backend with Spring Boot: A Comprehensive Guide
Introduction
When it comes to building a backend for a web application, scalability is a crucial factor to consider. A scalable backend is one that can handle an increasing amount of traffic, data, and user interactions without a significant decrease in performance. Spring Boot is a popular Java framework that provides a robust and flexible way to build scalable backends. In this article, we will explore the key concepts and techniques for building a scalable backend with Spring Boot.
Designing a Scalable Architecture
Before we dive into the nitty-gritty of Spring Boot, it's essential to understand the fundamentals of a scalable architecture. A scalable architecture is designed to handle an increasing load by efficiently utilizing resources, minimizing bottlenecks, and providing a flexible and modular design. Here are some key principles to consider:
- Separation of Concerns: Break down your application into smaller, independent components that communicate with each other. This allows for easier maintenance, scalability, and fault tolerance.
- Load Balancing: Distribute incoming traffic across multiple instances of your application to prevent any single instance from becoming a bottleneck.
- Caching: Implement caching mechanisms to reduce the load on your database and improve response times.
- Database Scaling: Design your database to scale horizontally or vertically, depending on your needs.
Building a Scalable Backend with Spring Boot
Now that we have a solid understanding of the principles of a scalable architecture, let's explore how to implement them using Spring Boot. Here are some key features and techniques to consider:
- Auto-Configuration: Spring Boot provides auto-configuration features that simplify the setup and configuration of your application. This includes features like embedded Tomcat, Hibernate, and caching.
- Microservices: Spring Boot is well-suited for building microservices-based architectures. Each microservice can be developed, deployed, and scaled independently, allowing for greater flexibility and scalability.
- Spring Cloud: Spring Cloud provides a set of tools and libraries that simplify the development of cloud-native applications. This includes features like service discovery, circuit breakers, and load balancing.
Scaling with Spring Data
Spring Data is a key component of the Spring ecosystem that provides a simple and consistent way to access data from various sources. Here are some key features and techniques to consider:
- Repository Pattern: Spring Data provides a repository pattern that simplifies data access and reduces boilerplate code.
- Query Methods: Spring Data provides query methods that allow you to define custom queries using a simple and intuitive API.
- Paging and Sorting: Spring Data provides built-in support for paging and sorting, making it easier to handle large datasets.
Scaling with Spring Messaging
Spring Messaging provides a simple and powerful way to build scalable messaging systems. Here are some key features and techniques to consider:
- Message Queue: Spring Messaging provides support for message queues like RabbitMQ, Apache Kafka, and Amazon SQS.
- Message Broker: Spring Messaging provides support for message brokers like Apache Kafka and RabbitMQ.
Case Study: Building a Scalable E-commerce Backend with Spring Boot
Let's put the concepts and techniques we've discussed into practice by building a scalable e-commerce backend with Spring Boot. Here's a hypothetical scenario:
- We're building an e-commerce application that sells products online.
- We expect a high volume of traffic and sales, especially during peak seasons.
- We need to ensure that our application can handle the load and provide a seamless user experience.
Here's a high-level overview of the architecture we might use:
- Frontend: We'll use a modern frontend framework like React or Angular to build the user interface.
- Backend: We'll use Spring Boot to build the backend, with a focus on scalability and performance.
- Database: We'll use a distributed database like Apache Cassandra or MongoDB to store product information and order data.
- Message Queue: We'll use a message queue like RabbitMQ or Apache Kafka to handle order processing and payment processing.
Implementing Load Balancing and Clustering
To distribute incoming traffic across multiple instances of our application, we'll use load balancing. We can use a load balancer like HAProxy or NGINX to distribute traffic across multiple instances of our application.
Clustering is another essential technique for scaling our backend. By grouping multiple instances of our application together, we can ensure that our application remains available even if one instance fails.
Using Caching and Content Delivery Networks (CDNs)
Caching is an effective technique for reducing the load on our database and improving response times. We can use caching mechanisms like Redis or Memcached to store frequently accessed data.
Content Delivery Networks (CDNs) are another effective technique for reducing the load on our backend. By caching static assets like images and videos at edge locations closer to our users, we can reduce latency and improve the overall user experience.
Database Scaling and Sharding
As our user base grows, we need to ensure that our database can handle the increased traffic and data volume. We can use database sharding to horizontally partition our data across multiple databases, each serving a specific subset of our users.
Another effective technique for scaling our database is to use a distributed database like Apache Cassandra or MongoDB. These databases are designed to handle large volumes of data and provide high availability and scalability.
Monitoring and Analytics
To ensure that our backend is performing optimally, we need to monitor and analyze its performance. We can use tools like Prometheus and Grafana to monitor our application's performance and identify bottlenecks.
Conclusion
Building a scalable backend with Spring Boot requires careful planning, design, and implementation. By following the principles of a scalable architecture and leveraging the features and techniques provided by Spring Boot, we can build a robust and performant backend that can handle an increasing load and provide a seamless user experience. Remember to consider the key principles of separation of concerns, load balancing, caching, and database scaling, and to take advantage of Spring Boot's auto-configuration, microservices, and Spring Cloud features. With the right design and implementation, we can build a scalable backend that meets the needs of our application and our users.
Final Thoughts
In this article, we've explored the key concepts and techniques for building a scalable backend with Spring Boot. We've discussed the principles of a scalable architecture, the features and techniques provided by Spring Boot, and the importance of load balancing, caching, and database scaling. We've also put these concepts into practice by building a scalable e-commerce backend with Spring Boot. By following the principles and techniques outlined in this article, we can build a robust and performant backend that can handle an increasing load and provide a seamless user experience.