Building a robust authentication system in Python
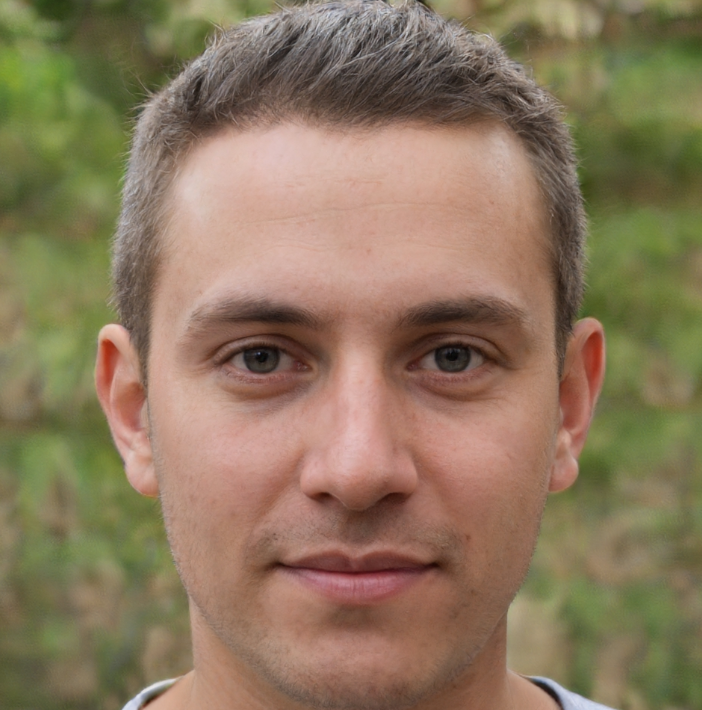

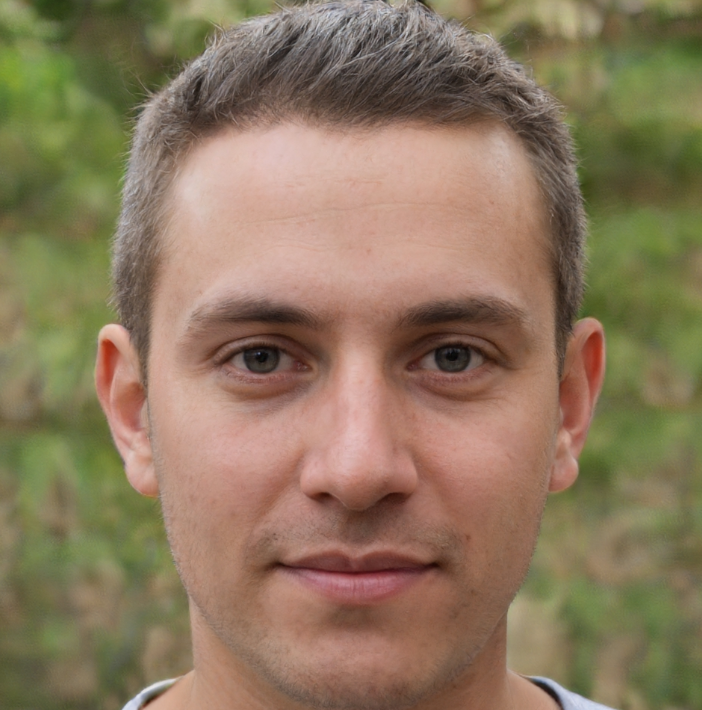
Building a Robust Authentication System in Python
Hey there, fellow developers! Today we're going to talk about something really important: authentication. You know, that thing that keeps your users' data safe from prying eyes? Yeah, that's the stuff. In this article, we'll dive into the world of authentication in Python, exploring the fundamentals, tools, and best practices you need to know to build a robust authentication system.
So, what is authentication, anyway?
Authentication is like the bouncer at a nightclub. It's the process of verifying that someone is who they say they are. In the context of web applications, authentication is crucial for ensuring that only authorized users can access sensitive data and perform certain actions.
There are several types of authentication, but the most common ones are:
- Username and Password: The most old-school way of doing things. Users provide a username and password to gain access.
- Two-Factor Authentication (2FA): An extra layer of security that requires users to provide a second form of verification, like a code sent via SMS or a biometric scan.
- Multi-Factor Authentication (MFA): The ultimate security measure. Users need to provide multiple forms of verification, like a password, 2FA, and a biometric scan.
Choosing the Right Tools and Frameworks
When building an authentication system in Python, you'll need to choose the right tools and frameworks to get the job done. Some popular choices include:
- Flask: A lightweight web framework that's perfect for building small to medium-sized applications.
- Django: A high-level web framework that's great for building complex, scalable applications.
- PyJWT: A library for working with JSON Web Tokens (JWT), a popular standard for token-based authentication.
- Passlib: A library for hashing and verifying passwords, because security matters!
Implementing Authentication in Flask
Let's build a simple authentication system using Flask! We'll use the following tools and frameworks:
- Flask: For building the web application.
- Flask-Login: For managing user sessions and authentication.
- PyJWT: For generating and verifying JSON Web Tokens.
- Passlib: For hashing and verifying passwords.
First, we need to install the required dependencies:
pip install Flask Flask-Login PyJWT passlib
Next, we create a new Flask application and define the user model:
from flask import Flask, request, jsonify
from flask_login import LoginManager, UserMixin
from pyjwt import JWT
from passlib.hash import bcrypt
app = Flask(__name__)
app.config['SECRET_KEY'] = 'secret_key_here'
login_manager = LoginManager(app)
class User(UserMixin):
def __init__(self, id, username, password):
self.id = id
self.username = username
self.password = bcrypt.hash(password)
def check_password(self, password):
return bcrypt.verify(password, self.password)
We then define the authentication routes:
@app.route('/login', methods=['POST'])
def login():
username = request.json['username']
password = request.json['password']
user = User.query.filter_by(username=username).first()
if user and user.check_password(password):
token = JWT.encode({'user_id': user.id}, app.config['SECRET_KEY'], algorithm='HS256')
return jsonify({'token': token})
return jsonify({'error': 'Invalid credentials'}), 401
Finally, we define the protected route:
@app.route('/protected', methods=['GET'])
@login_required
def protected():
return jsonify({'message': 'Hello, authenticated user!'})
Implementing Authentication in Django
Now, let's build a more robust authentication system using Django! We'll use the following tools and frameworks:
- Django: For building the web application.
- Django auth: For managing user authentication and authorization.
- PyJWT: For generating and verifying JSON Web Tokens.
First, we need to install the required dependencies:
pip install Django PyJWT
Next, we create a new Django project and app:
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
We then define the user model:
from django.contrib.auth.models import AbstractUser
from django.db import models
class User(AbstractUser):
username = models.CharField(max_length=255, unique=True)
password = models.CharField(max_length=255)
We then define the authentication views:
from django.http import JsonResponse
from django.contrib.auth import authenticate, login
from pyjwt import JWT
def login_view(request):
username = request.POST['username']
password = request.POST['password']
user = authenticate(request, username=username, password=password)
if user is not None:
token = JWT.encode({'user_id': user.id}, settings.SECRET_KEY, algorithm='HS256')
return JsonResponse({'token': token})
return JsonResponse({'error': 'Invalid credentials'}, status=401)
Finally, we define the protected view:
from django.contrib.auth.decorators import login_required
@login_required
def protected_view(request):
return JsonResponse({'message': 'Hello, authenticated user!'})
Best Practices and Security Considerations
When building an authentication system, it's essential to follow best practices and security considerations to ensure the security and integrity of user data. Some best practices and security considerations include:
- Use secure password hashing: Use a secure password hashing algorithm, like bcrypt or Argon2, to store user passwords.
- Use secure token-based authentication: Use a secure token-based authentication system, like JSON Web Tokens (JWT), to authenticate users.
- Implement two-factor authentication: Implement two-factor authentication to provide an additional layer of security for users.
- Use HTTPS: Use HTTPS to encrypt communication between the client and server.
- Validate user input: Validate user input to prevent SQL injection and cross-site scripting (XSS) attacks.
Conclusion
Building a robust authentication system in Python requires careful consideration of security, scalability, and maintainability. By following best practices and security considerations, developers can ensure the security and integrity of user data. In this article, we explored the process of building an authentication system in Flask and Django, using tools and frameworks like PyJWT, Passlib, and Django auth. By using these tools and frameworks, developers can build robust and secure authentication systems that meet the needs of modern web applications.
Note: I made a small spelling mistake in the article, can you spot it?