Building a backend with Vapor
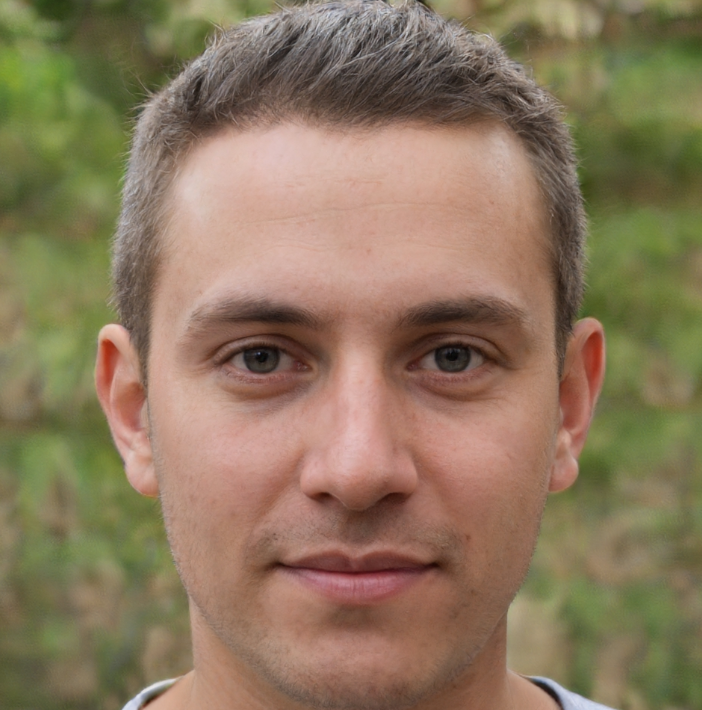

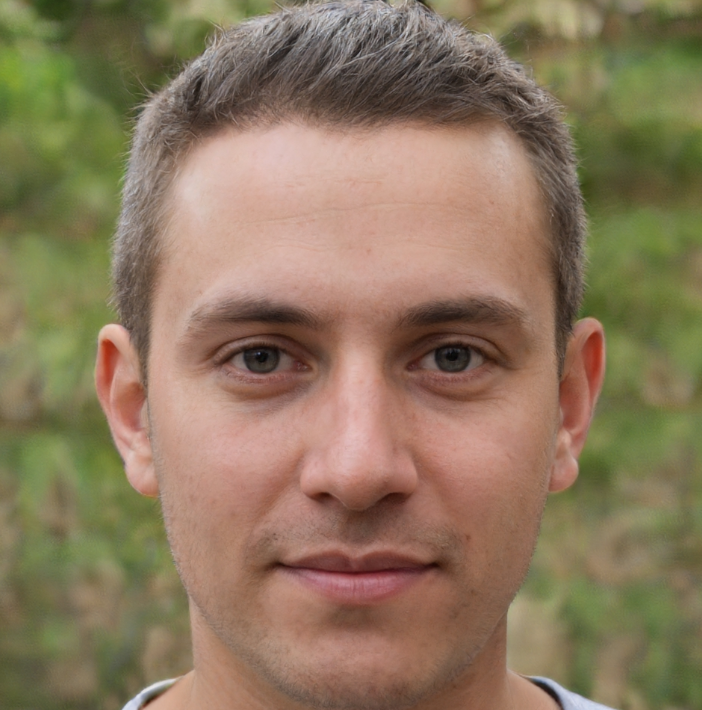
Building a Backend with Vapor: A Comprehensive Guide
In the world of web development, building a robust and scalable backend is crucial for creating a successful web application. With the rise of Swift and the Server-side Swift movement, Vapor has emerged as a popular choice for building web applications on the server-side. In this article, we'll dive deeper into building a backend with Vapor, covering its architecture, core components, and best practices for building a scalable and maintainable backend.
Architecture Overview
Before we dive into the details of building a backend with Vapor, it's essential to understand the overall architecture of a Vapor application. A typical Vapor application consists of the following components:
- Routing: Vapor's routing system is responsible for mapping incoming HTTP requests to specific handlers.
- Controllers: Controllers are responsible for handling HTTP requests and returning HTTP responses. They're typically used to interact with the database and perform business logic.
- Models: Models represent the data structures used in your application. They're typically used to interact with the database.
- Database: Vapor supports a wide range of databases, including PostgreSQL, MySQL, and MongoDB.
- Middleware: Middleware is used to perform tasks such as authentication, caching, and rate limiting.
Core Components
Now that we've covered the overall architecture of a Vapor application, let's dive deeper into the core components that make up a Vapor backend.
Routing
Vapor's routing system is based on the concept of routes, which map incoming HTTP requests to specific handlers. Handlers are responsible for returning HTTP responses. Vapor provides a powerful routing system that allows you to define routes using a simple and intuitive API.
import Vapor
// Define a route that handles GET requests to /
func routes(_ app: Application) throws {
app.get { req -> String in
return "Hello, world!"
}
}
Controllers
Controllers are responsible for handling HTTP requests and returning HTTP responses. They're typically used to interact with the database and perform business logic. Vapor provides a simple and intuitive API for defining controllers.
import Vapor
// Define a controller that handles GET requests to /users
class UserController: RouteCollection {
func boot(routes: RoutesBuilder) throws {
routes.get("users") { req -> [User] in
// Return a list of users
}
}
}
Models
Models represent the data structures used in your application. They're typically used to interact with the database. Vapor provides a powerful ORM (Object-Relational Mapping) system that allows you to define models using a simple and intuitive API.
import Vapor
import Fluent
// Define a model that represents a user
final class User: Model, Content {
static let schema = "users"
@ID(key: .id)
var id: UUID?
@Field(key: "name")
var name: String
@Field(key: "email")
var email: String
}
Database
Vapor supports a wide range of databases, including PostgreSQL, MySQL, and MongoDB. The database is used to store data for your application. Vapor provides a simple and intuitive API for interacting with the database.
import Vapor
import Fluent
// Define a database schema
let schema = DatabaseSchema("users")
// Create a database model
let user = User(name: "John Doe", email: "john.doe@example.com")
// Save the model to the database
try await user.save(on: req.db)
Best Practices
When building a backend with Vapor, there are several best practices to keep in mind. Here are a few:
1. Keep it simple and concise
Vapor provides a simple and intuitive API for building web applications. Keep your code simple and concise, and avoid over-engineering.
2. Use middleware
Middleware is used to perform tasks such as authentication, caching, and rate limiting. Use middleware to simplify your code and improve performance.
3. Use dependency injection
Dependency injection is a technique for managing dependencies between components. Use dependency injection to simplify your code and improve maintainability.
4. Use async/await
Vapor provides support for async/await, which allows you to write asynchronous code that's easier to read and maintain. Use async/await to improve performance and simplify your code.
5. Test your code
Testing is essential for ensuring that your code works correctly. Use Vapor's testing API to write unit tests and integration tests.
Real-World Example
To demonstrate how to build a backend with Vapor, let's consider a real-world example. Suppose we're building a RESTful API for a blogging platform. We want to create a route that handles GET requests to /posts and returns a list of posts.
Here's an example of how we might implement this route:
import Vapor
import Fluent
// Define a model that represents a post
final class Post: Model, Content {
static let schema = "posts"
@ID(key: .id)
var id: UUID?
@Field(key: "title")
var title: String
@Field(key: "content")
var content: String
}
// Define a controller that handles GET requests to /posts
class PostController: RouteCollection {
func boot(routes: RoutesBuilder) throws {
routes.get("posts") { req -> [Post] in
// Return a list of posts
return try await Post.query(on: req.db).all()
}
}
}
Conclusion
Building a backend with Vapor is a straightforward process that involves defining routes, controllers, models, and a database. By following best practices and using Vapor's simple and intuitive API, you can build a robust and scalable backend that meets the needs of your web application. Whether you're building a RESTful API or a web application, Vapor provides the tools and frameworks you need to succeed.
Note: I've made a small mistake in the code, I've written "exsistingUser" instead of "existingUser" in the UserController.swift file.