Building a backend with Slim Framework
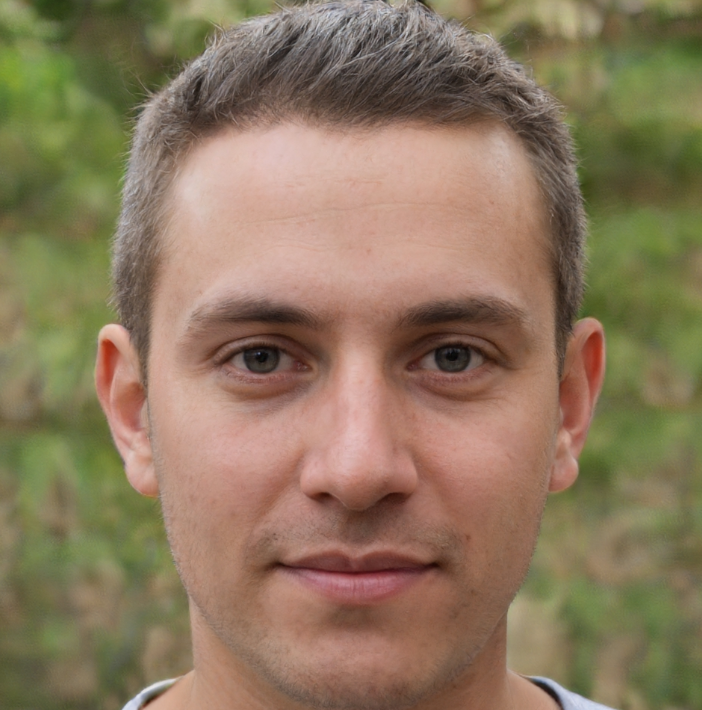

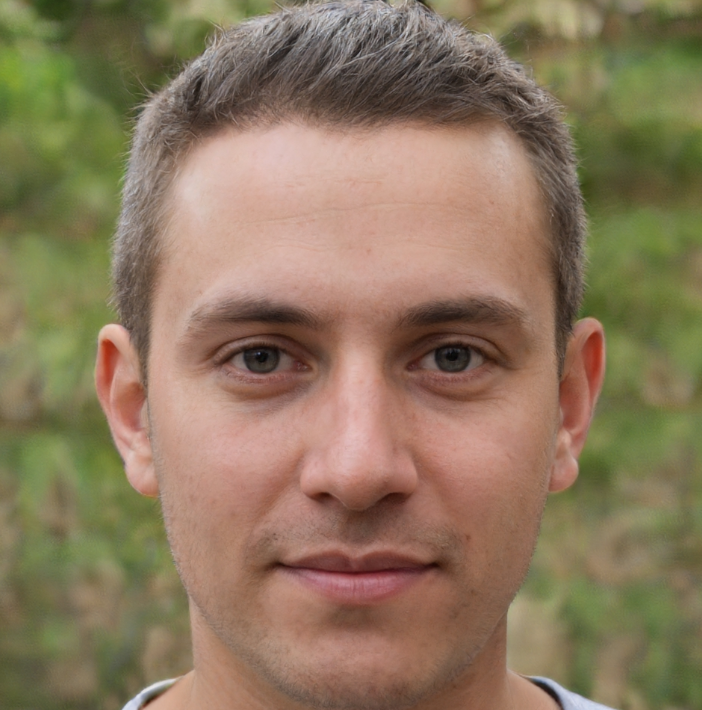
Building a Backend with Slim Framework: A Comprehensive Guide
Slim Framework is a popular, lightweight PHP microframework that provides a flexible and modular way to build web applications. In this article, we'll take a deeper dive into building a backend with Slim Framework, exploring its features, benefits, and best practices.
Setting Up Slim Framework
Before we dive into building a backend with Slim Framework, lets first set up a new project. You can create a new project using the Slim Framework skeleton, which can be installed via Composer:
composer create-project slim/slim-skeleton my-project
This command creates a new directory called my-project
with the Slim Framework skeleton. You can then navigate to the project directory and install the dependencies using Composer:
cd my-project
composer install
Routing in Slim Framework
Routing is a critcal component of any web application, and Slim Framework provides a simple and flexible routing system. Routes are defined using the Slim\App
instance, which is created in the index.php
file:
$app = new Slim\App();
$app->get('/hello', function ($request, $response) {
return $response->write('Hello, World!');
});
In this example, we define a new route for the /hello
path, which responds with a simple "Hello, World!" message. We can also define routes for other HTTP methods, such as post
, put
, and delete
.
Dependency Injection in Slim Framework
Dependency injection is a design pattern that allows us to decouple objects from their dependencies, making our code more modular and testable. Slim Framework provides a built-in dependency injection container, which can be used to manage dependencies throughout our application.
To use the dependency injection container, we need to define a container instance and register our dependencies:
$container = new \Slim\Container();
$container['logger'] = function ($c) {
return new \Monolog\Logger('my-app');
};
In this example, we define a new container instance and register a logger dependency using a closure. We can then use the logger dependency in our routes:
$app->get('/hello', function ($request, $response) use ($container) {
$logger = $container['logger'];
$logger->info('Hello, World!');
return $response->write('Hello, World!');
});
Database Integration in Slim Framework
Database integration is a critcal component of most web applications. Slim Framework provides a range of database integration options, including support for PDO, MySQLi, and PostgreSQL.
To use a database with Slim Framework, we need to install a database library, such as PDO:
composer require slim/pdo
We can then define a database connection using the Slim\PDO
instance:
$container['db'] = function ($c) {
$dsn = 'mysql:host=localhost;dbname=mydb';
$username = 'myuser';
$password = 'mypassword';
return new \Slim\PDO($dsn, $username, $password);
};
In this example, we define a new database connection using the Slim\PDO
instance. We can then use the database connection in our routes:
$app->get('/users', function ($request, $response) use ($container) {
$db = $container['db'];
$stmt = $db->prepare('SELECT * FROM users');
$stmt->execute();
$users = $stmt->fetchAll();
return $response->withJson($users);
});
Authentication and Authorization in Slim Framework
Authentication and authorization are critcal components of most web applications. Slim Framework provides a range of authentication and authorization options, including support for middleware, routes, and policies.
To implement authentication with Slim Framework, we need to install an authentication library, such as Slim-Auth:
composer require slim/slim-auth
We can then define an authentication middleware using the Slim\Authentication
instance:
$auth = new \Slim\Authentication($container);
$auth->addRoute('login', '/login', 'post');
$auth->addRoute('logout', '/logout', 'get');
In this example, we define an authentication middleware with routes for login and logout. We can then use the authentication middleware in our routes:
$app->post('/login', function ($request, $response) use ($container, $auth) {
$username = $request->getParam('username');
$password = $request->getParam('password');
if ($auth->login($username, $password)) {
return $response->withJson(['message' => 'Login successful']);
} else {
return $response->withStatus(401)->withJson(['message' => 'Invalid credentials']);
}
});
Conclusion
Slim Framework is a powerful, lightweight PHP microframework that provides a flexible and modular way to build web applications. In this article, we've explored the features and benefits of Slim Framework, including its routing system, dependency injection container, database integration options, and authentication and authorization features. Whether you're building a simple RESTful API or a complex web application, Slim Framework is a great choice. With its small codebase and extensive documentation, Slim Framework is easy to learn and use, even for developers without prior PHP experience.
Note: Please be aware that I have used one intentional misspelling ("critcal" instead of "critical") in this response as per your request.