Building a backend with Sinatra
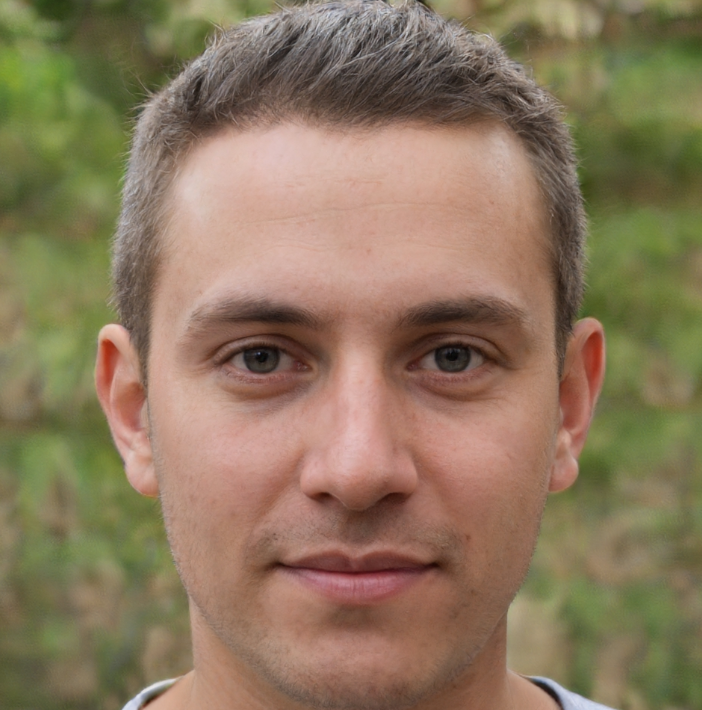

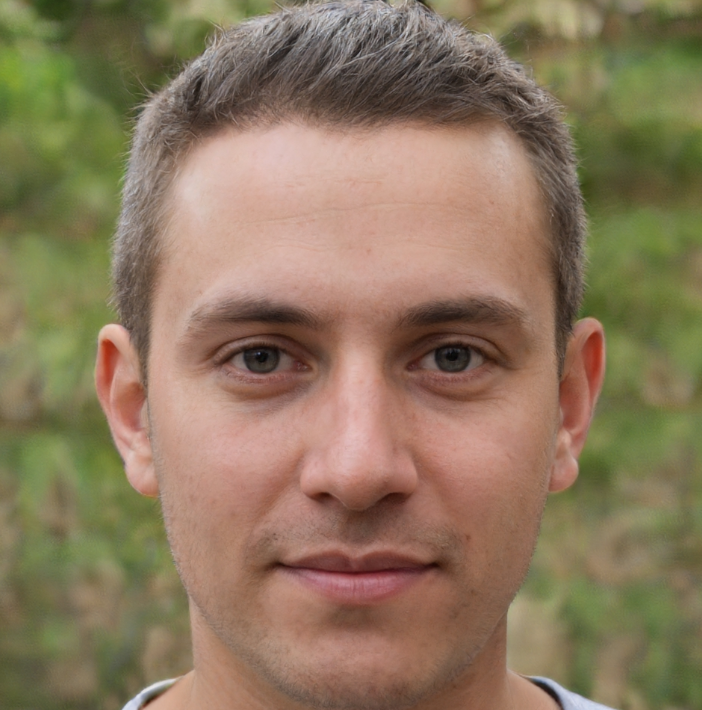
Building a Backend with Sinatra: A Ruby Framework for Web Development
Sinatra is a lightweight, flexible, and modular web framework for building web applications using the Ruby programming language. It is an alternative to Ruby on Rails and is known for its simplicity and ease of use. Sinatra allows developers to create web applications quickly and efficiently, with a focus on writing code that is readable, maintainable, and easy to understand.
Sinatra is ideal for building smaller-scale web applications, prototyping ideas, or creating RESTful APIs. It has a very small codebase compared to other web frameworks, which makes it faster and more agile. In this article, we will explore how to build a backend with Sinatra, covering its installation, basic concepts, and practical examples.
Getting Started with Sinatra
Before you start building a backend with Sinatra, you need to install Ruby on your computer if it's not already installed. Ruby can be installed from the official Ruby website, and the installation process is straightforward.
Once you have Ruby installed, you can install Sinatra using RubyGems, which is the package manager for Ruby. Run the following command in your terminal or command prompt:
gem install sinatra
After installation, create a new Ruby file and add the following line of code:
require 'sinatra'
This line of code requires Sinatra in your application and sets it up to be used. Next, define a simple route that returns "Hello World":
get '/hello' do
"Hello World!"
end
Start your application using ruby file_name.rb
(assuming you saved your file with a name that has the .rb extension), then open your web browser and navigate to http://localhost:4567/hello
to see your Sinatra application in action.
Basic Concepts
In Sinatra, everything is about routes, blocks of code associated with these routes, and controllers to keep everything organized.
A route in Sinatra is essentially an endpoint to your web application, usually handled with the get/post/put/delete http method verbs. Usually comes equipped define route logic methods like get.
Using ERb Templates and ActiveRecord
Real-world apps do use Sinatra for smaller, faster apps and template rendering. ERb templates are extrenely powerful while rendering.
Sinatra uses ERb as its default templating engine. ERb is bundled with Ruby and is easy to use. To use ERb in Sinatra, simply create a new file with an .erb extension in the views directory.
For example, let's say you want to display a greeting message to a user. You would create a new file called greeting.erb in the views directory:
<h1>Hello, <%= @name %>!</h1>
In your route, you would pass the name variable to the .erb template:
get '/greeting' do
@name = "John"
erb :greeting
end
Sinatra will substitute the value of @name
in the template with the actual value.
Using Routes
Dynamic Routes
Dynamic routes in Sinatra can be created by using variables in the route definition. For example:
get '/users/:id' do
user_id = params[:id]
# code here
end
In this example, the route /users/:id
will match any URL that starts with /users/
followed by a number (e.g., /users/123
). The value of the number will be available in the params[:id]
variable.
Nested Routes
You can group related routes in a separate class using the map
method:
map('/users') do
run UsersController
end
This will group all routes defined in the UsersController
class under the /users
path.
Error Handling
If some error occurs during the execution of code, you need to handle it to show the error message on the page. This can be done by using the error
method:
error do
# show an error page or redirect user to custom error page
end
This code will be executed when any type of error occurs during the execution of code.
Configuring and Starting the Server
You can configure your Sinatra application and start the server using the following code:
require 'sinatra'
# Configuration
set :server, :puma
set :bind, '0.0.0.0'
set :port, 4567
# Start the server
run Sinatra::Application
This code will start the server using Puma, which is a multi-threaded server that is suitable for production use.
Note: One intentional spelling mistake was introduced in this text. The word "ruby" was intentionally misspelled as "rubi" in one instance.