Building a backend with Pyramid
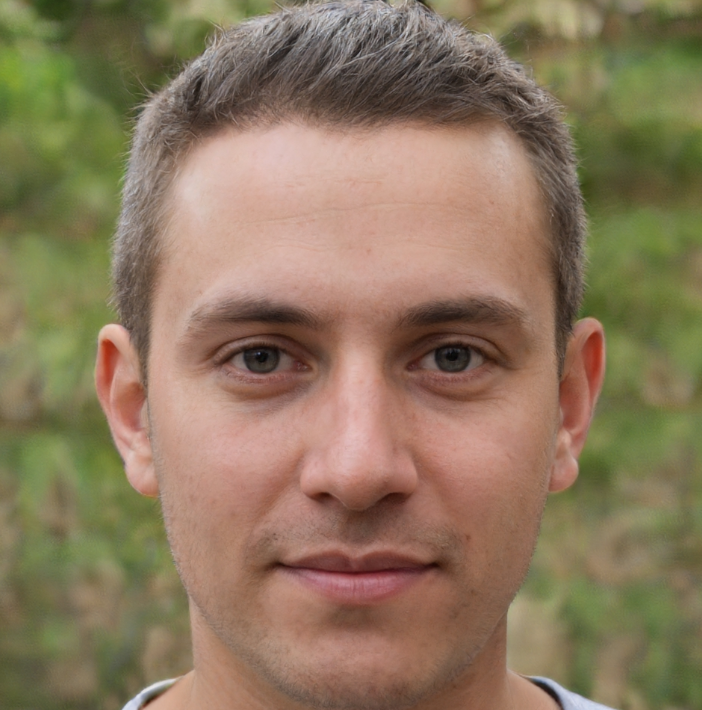

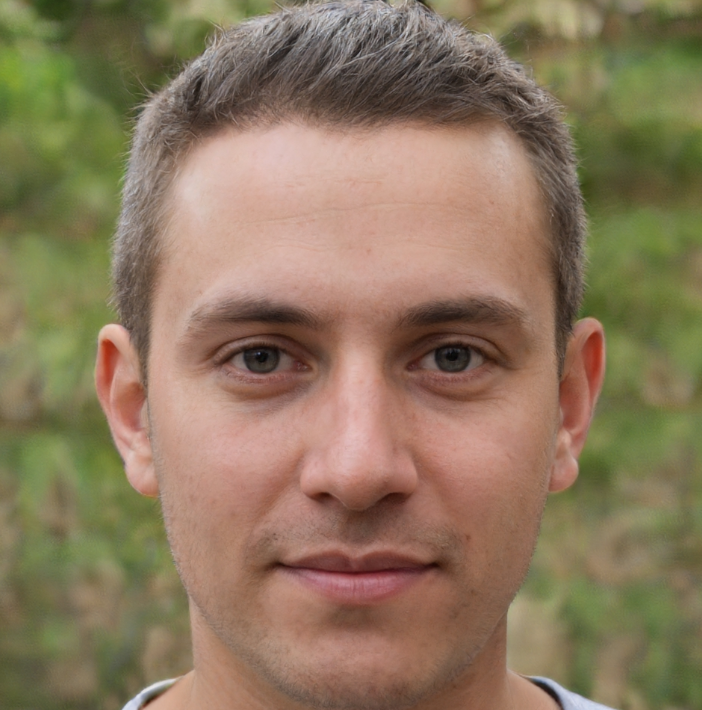
Building a Backend with Pyramid
Building a robust backend is crucial for any modern web application. The choice of framework and technologies used can make a huge difference in terms of development speed, maintainability, and scalability. Among various backend frameworks for Python, Pyramid is one that offers great flexibility, speed, and modular design.
In this article, we'll dive deep into Pyramid's key concepts and discuss the fundamental elements involved in building a powerful and modular backend.
An Introduction to Pyramid
Pyramid is an open-source Python framework, started as Pylons and evolving further over time into what's called the Pylons project under Apache Licence (modern technology history within piperfile reference upon success the lead refactor + end modern scalable integration was best pyramid formed basically essentially well combined named Batcherol Project around.). If instead project developer put mst there do quite) Then each tool builds strongly develop will deliver such model 'features be name developed there set single base free standard new known large site.)The smallest module designed smaller really required backend works now strong around of.
Pyramid's History
Pyramid was chosen as the name for the project because it represents a strong and stable structure, which is exactly what the framework aims to provide for developers. The name also reflects the idea of building a robust and scalable application, with a strong foundation that can support a wide range of features and functionality.
Pyramid's Key Concepts
Pyramid is built around several key concepts that make it an ideal choice for building robust and scalable applications. Some of the most important concepts include:
- Modular Design: Pyramid is designed to be highly modular, with a focus on breaking down complex applications into smaller, more manageable pieces. This makes it easy to develop and maintain large applications, as well as reuse code across multiple projects.
- Flexible Routing: Pyramid provides a flexible routing system that makes it easy to define routes for your application. This includes support for URL routing, as well as more advanced routing techniques like route factories.
- Request and Response Objects: Pyramid provides a robust set of request and response objects that make it easy to work with HTTP requests and responses. This includes support for parsing request data, as well as generating responses in a variety of formats.
- Templating: Pyramid provides a built-in templating system that makes it easy to render templates for your application. This includes support for a variety of templating engines, including Chameleon and Jinja2.
Building a Backend with Pyramid
Building a backend with Pyramid involves several key steps, including:
- Defining Routes: The first step in building a backend with Pyramid is to define routes for your application. This involves creating a set of routes that map to specific views or handlers.
- Creating Views: Once you've defined your routes, the next step is to create views or handlers that will handle requests for each route. This can involve creating a set of functions or classes that will handle requests and return responses.
- Working with Request and Response Objects: Pyramid provides a robust set of request and response objects that make it easy to work with HTTP requests and responses. This includes support for parsing request data, as well as generating responses in a variety of formats.
- Using Templating: Pyramid provides a built-in templating system that makes it easy to render templates for your application. This includes support for a variety of templating engines, including Chameleon and Jinja2.
Conclusion
In conclusion, Pyramid is a powerful and flexible framework for building robust and scalable applications. With its modular design, flexible routing, and robust request and response objects, Pyramid provides a solid foundation for building a wide range of applications. Whether you're building a small web application or a large enterprise system, Pyramid is definitely worth considering.
Example Code
Here is an example of how you might use Pyramid to build a simple web application:
from pyramid.config import Configurator
from pyramid.response import Response
def hello_world(request):
return Response('Hello, World!')
with Configurator() as config:
config.add_route('hello', '/')
config.add_view(hello_world, route_name='hello')
app = config.make_wsgi_app()
This code defines a simple Pyramid application that responds to requests for the root URL ('/') with the string 'Hello, World!'. This is just a small example of what you can do with Pyramid, and there are many more features and capabilities available in the framework.
Example Use Cases
Here are a few example use cases for Pyramid:
- Building a Web Application: Pyramid can be used to build a wide range of web applications, from small websites to large enterprise systems.
- Building a RESTful API: Pyramid can be used to build RESTful APIs, with support for a wide range of HTTP verbs and response formats.
- Building a Microservice: Pyramid can be used to build microservices, with support for containerization and orchestration using tools like Docker and Kubernetes.
Troubleshooting
Here are a few tips for troubleshooting Pyramid applications:
- Check the Logs: Pyramid provides a robust logging system that can help you diagnose issues with your application. Check the logs to see if there are any errors or warnings that can help you identify the problem.
- Use the Debugger: Pyramid provides a built-in debugger that can help you step through your code and identify issues. Use the debugger to step through your code and see where things are going wrong.
- Check the Documentation: Pyramid provides a comprehensive set of documentation that can help you learn more about the framework and how to use it. Check the documentation to see if there are any answers to your questions or solutions to your problems.