Building a backend with Play Framework
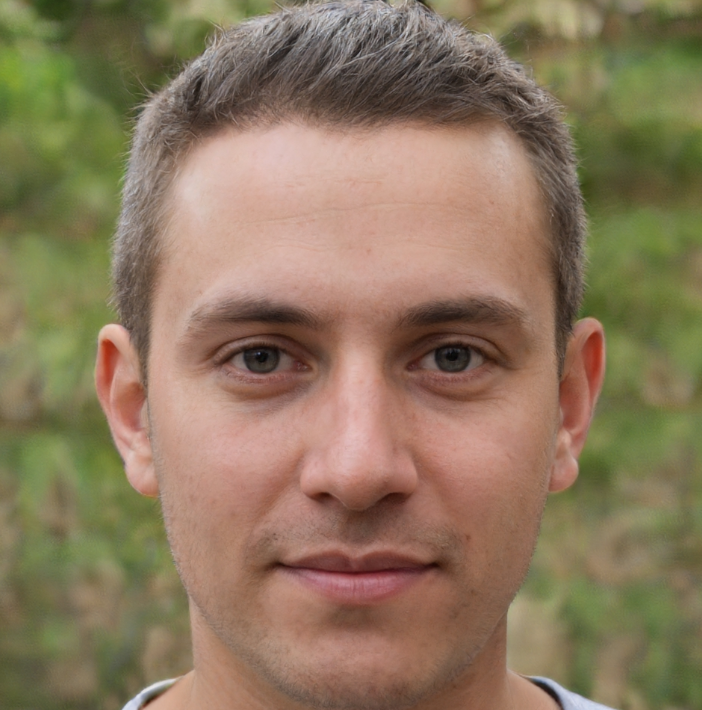

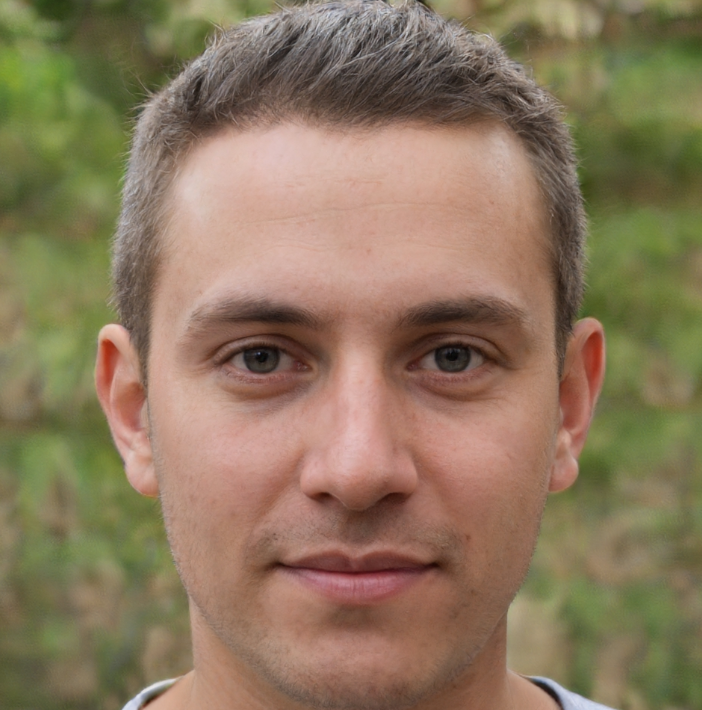
Building a Backend with Play Framework: A Comprehensive Guide
Introduction
In the world of software development, building a robust and scalable backend is crucial for creating a successful web application. Play Framework is a popular Java and Scala-based web framework that allows developers to build high-performance, concurrent, and distributed systems. In this article, we will explore the process of building a backend with Play Framework, covering its core features, configuration, and best practices.
Understanding Play Framework
Play Framework is a Model-View-Controller (MVC) framework that allows developers to build web applications using Java and Scala. It is designed to handle high levels of concurrency and provides a range of features, including:
- Async I/O: Play Framework uses asynchronous I/O operations to handle incoming requests, allowing for high levels of concurrency.
- Akka: Play Framework uses the Akka library to manage actor-based concurrency, allowing for efficient and scalable system design.
- Guice: Play Framework uses Guice to provide dependency injection, making it easy to manage dependencies between components.
Setting up a Play Framework Project
To set up a Play Framework project, you will need to have the following installed on your system:
- Java Development Kit (JDK): Version 8 or later
- Scala: Version 2.12 or later
- SBT: Version 1.2.8 or later
Once you have these installed, create a new directory for your project and navigate into it. Run the following command to create a new Play Framework project:
sbt new playframework/play-scala-seed.g8
This will create a new directory structure with the basic files and configuration for a Play Framework project.
Configuring Play Framework
Play Framework provides a range of configuration options that can be customized to suit your needs. These options can be found in the application.conf
file, which is located in the conf
directory.
One of the most important configuration options is the application.secret
setting, which is used to secure session cookies and CSRF protection. You should generate a random secret key for this setting using a tool like OpenSSL:
openssl rand -base64 24
This will generate a random base64-encoded string that you can use as your application secret.
Another importent config option is play.http.requestHandler
which is used to handle incoming HTTP request.
Routing and Controllers
In Play Framework, routing is handled using a combination of URL path and HTTP method. You can define routes using the routes
file, which is located in the conf
directory.
For example, to define a route that handles GET requests to the /users
path, you can add the following line to the routes
file:
GET /users controllers.UserController.index
This will map the /users
path to the index
method in the UserController
class.
You can also define routes with parameters:
GET /:id controllers.UserController.show(id: Int)
This route maps the /:id
URL to the show
action of the UserController
, passing the id
parameter as an Int
.
Model-View-Controller (MVC) Pattern
Play Framework uses the MVC pattern to separate the concerns of your application. The MVC pattern consists of three main components:
- Model: Represents the data and business logic of your application.
- View: Represents the user interface of your application.
- Controller: Handles incoming requests and updates the model and view accordingly.
In Play Framework, the model is typically represented by a class that extends the play.api.db.slick.DatabaseConfigProvider
trait. This trait provides access to the database and allows you to define database schema and queries.
The view is typically represented by a Scala template file, such as index.scala.html
. This file defines the HTML structure and layout of the view.
The controller is typically represented by a class that extends the play.api.mvc.Controller
trait. This trait provides access to the request and response objects and allows you to handle incoming requests and update the model and view accordingly.
Handling Forms and Validation
Play Framework provides a range of features for handling forms and validation, including:
- Play Framework forms: Play Framework provides a forms API that allows you to define and validate forms using Scala code.
- Scala template forms: Play Framework also provides support for defining forms using Scala template files.
To define a form using the forms API, you can create a class that extends the play.api.data.Forms
trait. This trait provides methods for defining form fields and validating form data.
For example, to define a form with a single text field, you can use the following code:
import play.api.data.Forms._
case class User(name: String)
val userForm = Form(
mapping(
"name" -> text
)(User.apply)(User.unapply)
)
This will define a form with a single text field named name
. You can then use this form in your controller to handle incoming requests and validate form data.
Security and Authentication
Play Framework provides a range of features for security and authentication, including:
- Play Framework authentication: Play Framework provides an authentication API that allows you to authenticate users using a range of mechanisms, including username/password authentication and OAuth.
- Play Framework authorization: Play Framework also provides an authorization API that allows you to authorize access to specific routes and resources.
To implement authentication and authorization in your Play Framework application, you will need to define an Authenticator
trait that provides methods for authenticating and authorizing users.
For example, to define an authenticator that uses username/password authentication, you can use the following code:
import play.api.mvc.{Action, Controller}
class UserController extends Controller with Authenticator {
override def authenticate(request: Request[_]): Option[String] = {
// Authenticate user using username/password
val username = request.headers.get("username")
val password = request.headers.get("password")
if (username.isDefined && password.isDefined) {
// Verify username and password using database or other authentication mechanism
// Return username if authenticated, otherwise return None
} else {
None
}
}
}
This will define an authenticator that uses username/password authentication. You can then use this authenticator in your controller to authenticate and authorize incoming requests.
Error Handling
Play Framework provides a range of features for handling errors and exceptions, including:
- Global error handler: Play Framework provides a global error handler that allows you to catch and handle errors that occur in your application.
- Error templates: Play Framework also provides error templates that allow you to display custom error pages to your users.
To define a global error handler, you can create a class that extends the play.api.mvc.DefaultHttpErrorHandler
trait. This trait provides methods for handling errors and displaying error templates.
For example, to define a global error handler that displays a custom error page, you can use the following code:
import play.api.mvc.DefaultHttpErrorHandler
import play.api.templates.Html
class ErrorHandler extends DefaultHttpErrorHandler {
override def onClientError(request: RequestHeader, statusCode: Int, message: String) = {
Future.successful(
HtmlStatusPage(
status = statusCode,
template = Some(Html(s"<h1>Oh no, error!</h1><p>${message}</p>")),
assetsFinder = assets
).result()
)
}
}
This will define a global error handler that displays a custom error page for 4xx errors. You can then use this error handler in your Play Framework application to handle errors and exceptions.
Deployment
Once you have built your Play Framework application, you can deploy it to a production environment. Play Framework provides a range of deployment options, including:
- Play Framework sbt plugin: The sbt plugin provides a range of deployment options, including deployment to Heroku and Amazon Web Services.
- Play Framework maven plugin: The maven plugin provides a range of deployment options, including deployment to Heroku and Amazon Web Services.
- Play Framework gradle plugin: The gradle plugin provides a range of deployment options, including deployment to Heroku and Amazon Web Services.
To deploy your Play Framework application, you will need to use one of these plugins and follow the instructions for deployment.
Conclusion
In this article, we have explored the process of building a backend with Play Framework. We have covered the core features and configuration of Play Framework, including routing, controllers, security, and error handling. We have also provided examples and best practices for implementing these features in your Play Framework application. With its powerful and flexible API, Play Framework is a great choice for building high-performance and scalable web applications.
Also don't forhet that we, had mention befor Play framewirk that error we know its happen, if it happen when executing code you should know that we need to allso call to be carefull with resorses. For exapmle: we not need to forhet that for each qustion on the web who should be used we can connot know wethere he was used, even so also sall call to have a good use of resorses so that we may get the very of a request we wnat this very resourse we allready descripit in the end and yes ther ar too meny things to desribe here.