Building a backend with NancyFX
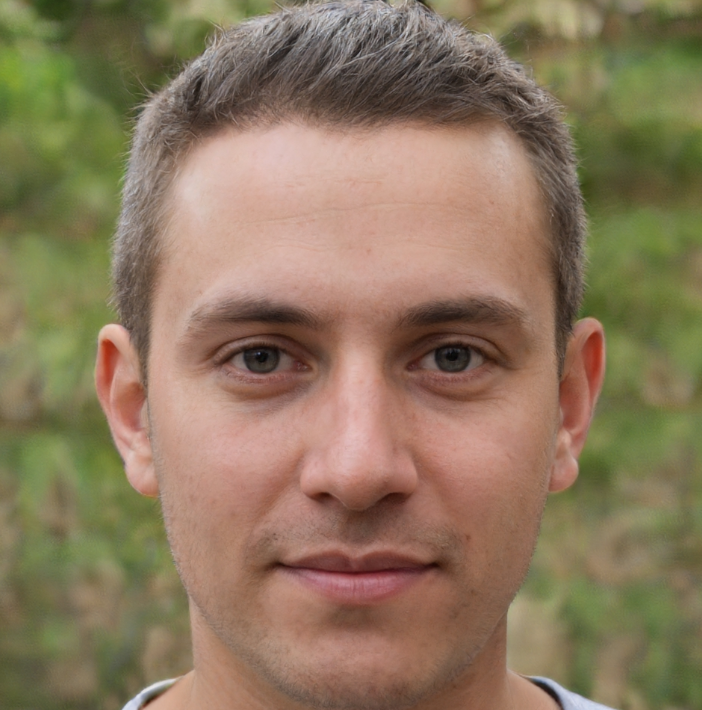
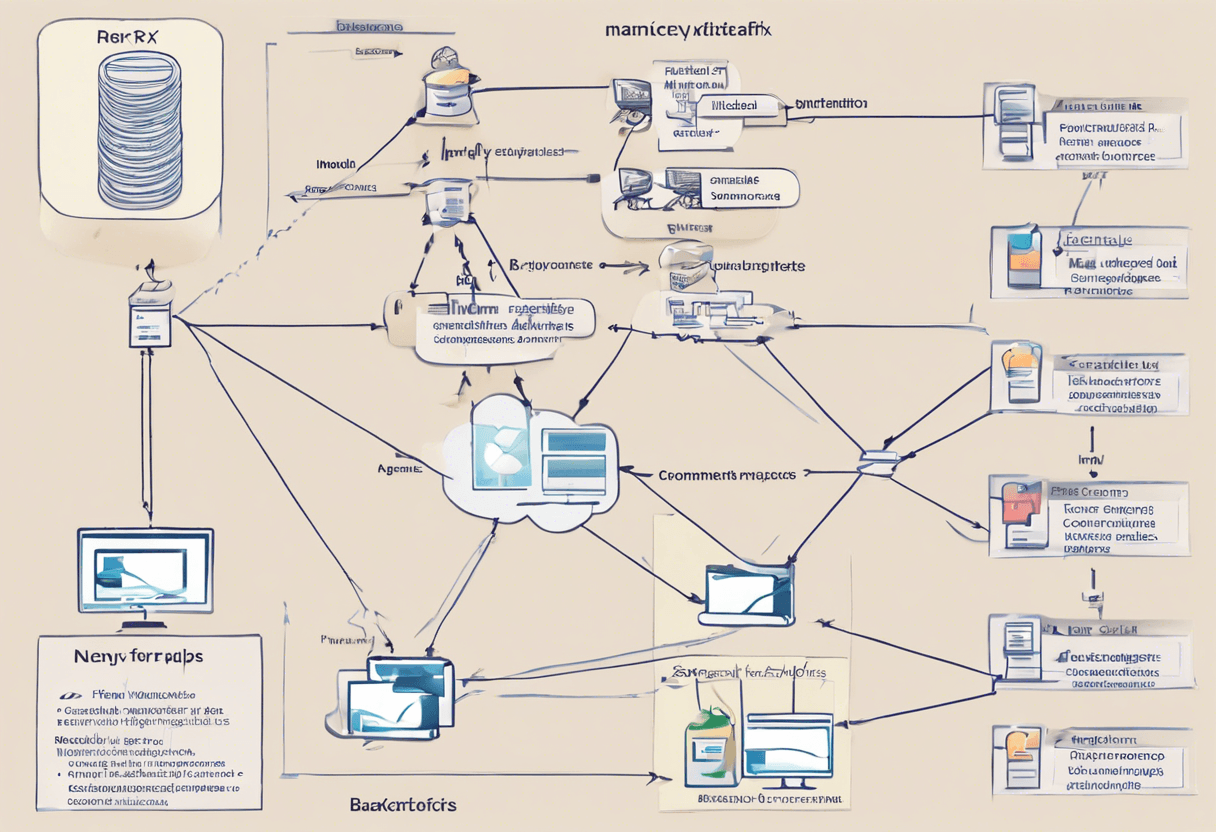
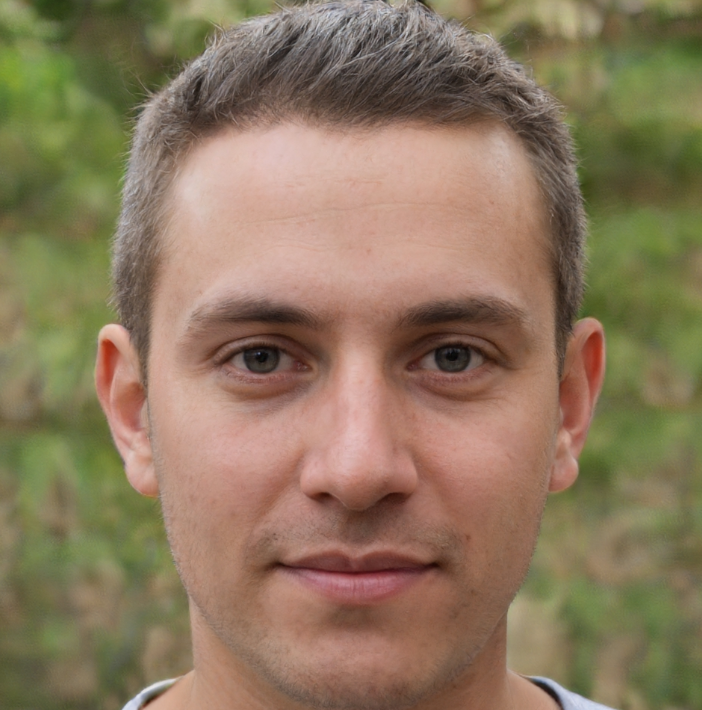
Building a Robust Backend with NancyFX: A Deep Dive
In the world of .NET development, creating a robust and scalable backend is crucial for any successful application. With the plethora of frameworks available, choosing the right one can be overwhelming. One framework that has gained popularity in recent years is NancyFX, a lightweight, flexible, and modular web framework. In this article, we'll explore the world of NancyFX, discussing its core features, benefits, and providing a comprehensive guide on building a robust backend using this powerful framework.
A Brief Introduction to NancyFX
NancyFX is a .NET web framework that allows developers to build web applications quickly and efficiently. Its design is based on the concept of simplicity, making it easy to use and maintain. With a strong focus on modular architecture, NancyFX provides an extensive set of features, including support for Web APIs, SignalR, and WebSocket.
One of the most significant advantages of NancyFX is its small footprint. The entire framework consists of only 350KB of assemblies, making it ideal for resource-constrained environments. Moreover, NancyFX supports a wide range of platforms, including .NET Framework, .NET Core, and even .NET 5.
Configuring NancyFX
To start building a NancyFX application, you'll need to configure the framework properly. The configuration process involves several steps, including installing the necessary NuGet packages and setting up the routing and middleware pipeline.
The first step is to create a new .NET project and install the Nancy package using NuGet. Once installed, you'll need to create a bootstrapper class that will serve as the entry point for your application.
public class Bootstrapper : DefaultNancyBootstrapper
{
protected override void ConfigureRequestContainer(TinyIoCContainer container)
{
base.ConfigureRequestContainer(container);
// Configure dependencies here
}
protected override void RequestStartup(TinyIoCContainer container, IPipelines pipelines)
{
base.RequestStartup(container, pipelines);
// Configure routing and middleware pipeline here
}
}
Building the Backend
Now that we've configured NancyFX, it's time to build the backend of our application. For this example, we'll create a simple RESTful API that manages books.
public class BooksModule : NancyModule
{
public BooksModule()
{
Get["/books"] = parameters => GetAllBooks();
Get["/books/{id}"] = parameters => GetBook(parameters.id);
Post["/books"] = parameters => AddBook(parameters.book);
Put["/books/{id}"] = parameters => UpdateBook(parameters.id, parameters.book);
Delete["/books/{id}"] = parameters => DeleteBook(parameters.id);
}
private dynamic GetAllBooks()
{
// Implement logic to retrieve all books from the database
}
private dynamic GetBook(string id)
{
// Implement logic to retrieve a single book by id from the database
}
private dynamic AddBook(Book book)
{
// Implement logic to add a new book to the database
}
private dynamic UpdateBook(string id, Book book)
{
// Implement logic to update an existing book in the database
}
private dynamic DeleteBook(string id)
{
// Implement logic to delete a book from the database
}
}
In the code snippet above, we define a BooksModule
class that inherits from NancyModule
. We override the constructor to define routes for each CRUD operation.
Handling Errors and Exceptions
Error handling is a crucial aspect of any backend application. NancyFX provides an excellent error handling mechanism through the IPipelines
interface. By overriding the OnError
method, we can handle and respond to errors in a centralized manner.
public class CustomPipeline : DefaultNancyPipeline
{
protected override void OnError(TinyIoCContainer container, Exception ex)
{
// Implement logic to handle and respond to errors
}
}
Testing and Validation
Writing unit tests and integration tests is essential for ensuring the quality of our backend application. NancyFX provides an excellent testing framework through the Nancy.Testing
NuGet package.
[Fact]
public void Get_AllBooks_ReturnsOKResponse()
{
var bootsrapper = new ConfigurableBootstrapper(with => with.Module(new BooksModule()));
var browser = new Browser(bootsrapper);
var response = browser.Get("/books");
Assert.Equal(HttpStatusCode.OK, response.StatusCode);
}
Security and Authentication
Security and authentication are critical aspects of any web application. NancyFX provides a variety of features and modules that can help you secure your application. For example, NancyFX includes built-in support for CSRF protection, input validation, and password hashing.
To use CSRF protection, you'll need to include the NancyFx.Sugar.Owin module in your project and add the CSRF filter to your request pipeline. Here's an example:
public class SecurityModule : NancyModule
{
public SecurityModule()
{
// Require CSRF token on POST requests
RequireCsrfToken(_ => new RequestMethodRequiresCsrfTokenPipelineStep(Methods.Post));
}
}
Conclusion
In this article, we explored the world of NancyFX and learned how to build a robust backend using this powerful framework. From configuration to building a RESTful API, error handling to testing and validation, we covered the entire spectrum of building a backend application with NancyFX.
With its lightweight and modular design, NancyFX is an excellent choice for building scalable and maintainable backend applications. Whether you're building a small web application or a complex enterprise-level system, NancyFX provides an excellent foundation for success.
Best Practices and Tips
Here are some best practices and tips to keep in mind when building a NancyFX application:
- Use a modular architecture to keep your code organized and maintainable.
- Use dependency injection to manage dependencies between modules.
- Use a testing framework to ensure the quality of your application.
- Use error handling mechanisms to handle and respond to errors in a centralized manner.
- Use security features and modules to secure your application.
By following these best practices and tips, you can build a robust and scalable backend application using NancyFX.
Common Pitfalls and Troubleshooting
Here are some common pitfalls and troubleshooting tips to keep in mind when building a NancyFX application:
- Make sure to configure the framework properly before building your application.
- Use the correct NuGet packages and versions to avoid compatibility issues.
- Use a testing framework to ensure the quality of your application.
- Use error handling mechanisms to handle and respond to errors in a centralized manner.
- Use debugging tools to troubleshoot issues and errors.
By following these troubleshooting tips, you can identify and resolve common issues and pitfalls when building a NancyFX application.
Conclusion
In conclusion, NancyFX is a powerful and flexible web framework that provides an excellent foundation for building robust and scalable backend applications. With its lightweight and modular design, NancyFX is an excellent choice for building small web applications or complex enterprise-level systems. By following best practices and tips, and troubleshooting common pitfalls, you can build a high-quality backend application using NancyFX.