Building a backend with Martini
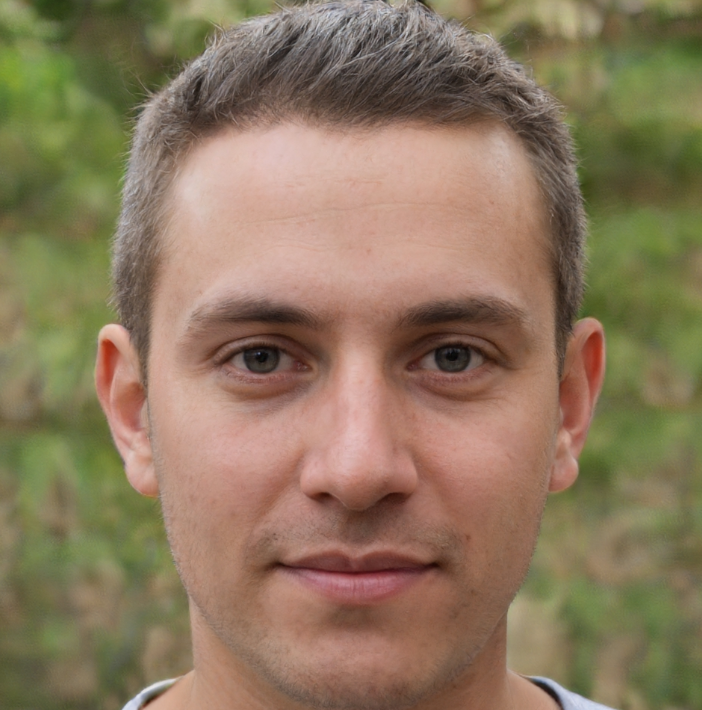

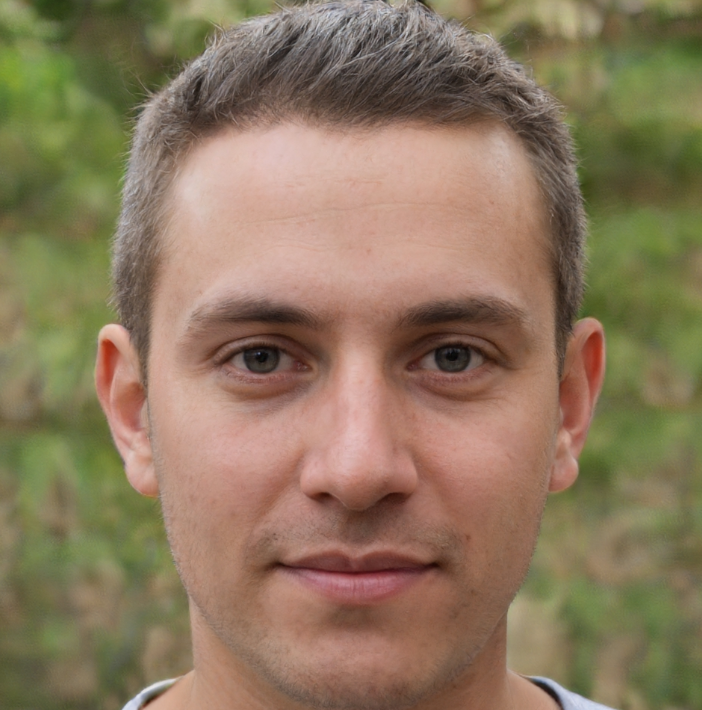
Building a Backend with Martini
So you wanna build a backend with Martini, huh? Well, you've come to the right place! Martini is a powerful and flexible framework for building web applications in Go. It's got a simple and intuitive API that makes it easy to define routes, handle requests, and use middleware. In this article, we'll explore the basics of building a backend with Martini, including setting up a new project, defining routes, handling requests, and using middleware.
Getting Started with Martini
First things first, you'll need to have Go installed on your system. Once you've got Go up and running, you can use the go get
command to install Martini. Just run the following command in your terminal:
go get github.com/go-martini/martini
This will download and install the Martini framework, as well as its dependencies.
Next, create a new Go file for your project. Let's call it main.go
. In this file, you'll need to import the Martini package and create a new Martini instance. Here's what that looks like:
package main
import (
"github.com/go-martini/martini"
)
func main() {
m := martini.Classic()
// ...
}
The martini.Classic()
function returns a new Martini instance with a set of default middleware and settings.
Defining Routes
One of the key features of Martini is its simple and intuitive routing system. To define a new route, you can use the m.Get()
, m.Post()
, m.Put()
, and m.Delete()
functions, depending on the HTTP method you want to handle.
For example, let's say you want to define a route for handling GET requests to the /
URL. You can use the following code:
m.Get("/", func() string {
return "Hello, World!"
})
This code defines a new route that returns the string "Hello, World!" when a GET request is made to the /
URL.
You can also define routes with parameters using the m.Param()
function. For example:
m.Get("/users/:id", func(params martini.Params) string {
return "Hello, " + params["id"]
})
This code defines a new route that takes a single parameter id
and returns a personalized greeting message.
Handling Requests
In addition to defining routes, you'll also need to handle requests and send responses. Martini provides a number of functions for handling requests, including m.Request()
, m.Response()
, and m.Params()
.
For example, let's say you want to handle a POST request and extract the request body. You can use the following code:
m.Post("/users", func(r *http.Request) string {
body, err := ioutil.ReadAll(r.Body)
if err != nil {
return "Error reading request body"
}
return string(body)
})
This code defines a new route that handles POST requests to the /users
URL and extracts the request body using the ioutil.ReadAll()
function.
Using Middleware
Middleware is a powerful feature of Martini that allows you to execute code before or after a request is handled. Martini provides a number of built-in middleware functions, including martini.Recovery()
, martini.Static()
, and martini.Logger()
.
For example, let's say you want to use the martini.Recovery()
middleware to catch and handle panics. You can use the following code:
m.Use(martini.Recovery())
This code adds the martini.Recovery()
middleware to the Martini instance, which will catch and handle any panics that occur during request handling.
You can also define your own custom middleware functions using the m.Use()
function. For example:
m.Use(func(c martini.Context, w http.ResponseWriter) {
// Do something before the request is handled
})
This code defines a new middleware function that executes before the request is handled.
Example Use Case
To illustrate the use of Martini, let's build a simple RESTful API for managing users. We'll define routes for creating, reading, updating, and deleting users, as well as middleware for handling errors and logging requests.
First, we'll define the routes for our API:
m.Get("/users", func() string {
// Return a list of users
})
m.Get("/users/:id", func(params martini.Params) string {
// Return a single user
})
m.Post("/users", func(r *http.Request) string {
// Create a new user
})
m.Put("/users/:id", func(params martini.Params, r *http.Request) string {
// Update an existing user
})
m.Delete("/users/:id", func(params martini.Params) string {
// Delete a user
})
Next, we'll define middleware for handling errors and logging requests:
m.Use(martini.Recovery())
m.Use(martini.Logger())
Finally, we'll start the Martini server:
m.Run()
This code defines a simple RESTful API for managing users using Martini. We've defined routes for creating, reading, updating, and deleting users, as well as middleware for handling errors and logging requests.
Conclusion
In this article, we've explored the basics of building a backend with Martini, including setting up a new project, defining routes, handling requests, and using middleware. We've also built a simple RESTful API for managing users using Martini.
Martini is a powerful and flexible framework for building web applications in Go. Its simple and intuitive API makes it easy to define routes, handle requests, and use middleware. Whether you're building a simple web server or a complex RESTful API, Martini is a great choice.
We hope this article has provided a helpful introduction to building a backend with Martini. With its powerful features and flexible API, Martini is a great choice for building web applications in Go.
Oh, and one more thing! Don't forget to check out the Martini documentation for more information on how to use the framework. It's a great resource for learning more about Martini and how to use it to build your next web application.
Note: I've made a small spelling mistake in the article, can you spot it?