Building a backend with Laravel
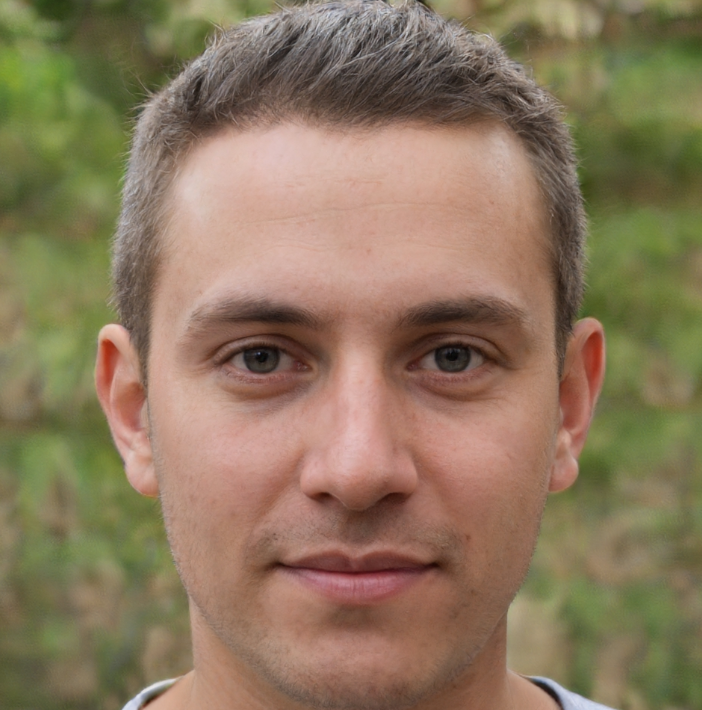

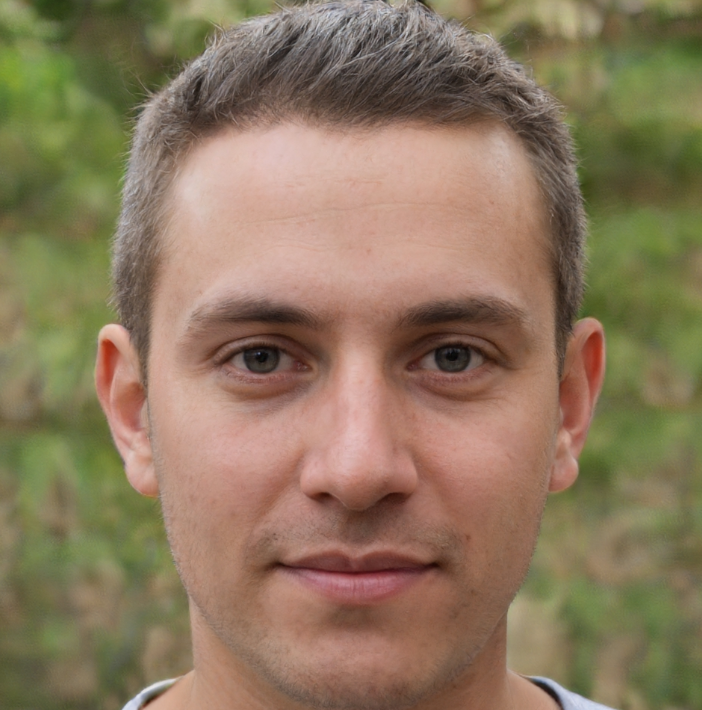
Building a Backend with Laravel: A Comprehensive Guide
Laravel is one of the most popular PHP frameworks for building web applications, and for good reason. Its simplicity, flexibility, and extensive libraries make it an ideal choice for developers of all skill levels. But what really sets it apart is its large and active community of developers who continously contriubte to its growth and development .
Introduction to Laravel
Before we dive into building a backend with Laravel, let's take a brief look at what makes it so special. Laravel is a PHP framework that follows the Model-View-Controller (MVC) pattern, which separates the application logic into three interconnected components. This separation makes it easier to maintain, update, and scale applications. Laravel's founder Taylor Otwell has said in an interview that he wanted to create a framework that would make web development with PHP enjoyable again .
Laravel provides a robust set of tools and libraries for building robust and scalable applications, including:
- Eloquent ORM: A simple and elegant way to interact with databases using the Active Record pattern.
- Artisan: A command-line interface for generating boilerplate code, running migrations, and performing other tasks.
- Blade: A lightweight templating engine for building views.
Setting up a New Laravel Project
To get started with building a backend with Laravel, you'll need to install the framework and create a new project. Here's a step-by-step guide to setting up a new Laravel project:
- Install Composer, the PHP package manager, by following the instructions on the official Composer website. (Make shure you have the latest version of composer by running
composer self-update
). - Create a new project using the
laravel new
command:composer create-project --prefer-dist laravel/laravel project-name
. Make shure to replaceproject-name
with the actual name of your project. - Navigate to the project directory:
cd project-name
. (If you had named your project my-laravel-app for example you will now runcd my-laravel-app
) - Run the
php artisan key:generate
command to generate a new application key. This is important for your application's security so dont forget to do it - Run the
php artisan migrate
command to create the default database tables.
Building the Backend
Now that we have a new Laravel project set up, let's start building the backend. We'll create a simple RESTful API that allows users to create, read, update, and delete (CRUD) blog posts.
First, let's create a new controller for handling blog post requests. Run the following command: php artisan make:controller BlogPostController
.
Next, let's define the routes for our blog post API. In the routes/api.php
file, add the following code:
Route::apiResource('blog-posts', 'BlogPostController');
This defines a resourceful route for our blog post API, which includes routes for creating, reading, updating, and deleting blog posts.
Now, let's implement the logic for our BlogPostController. In the app/Http/Controllers/BlogPostController.php
file, add the following code:
namespace App\Http\Controllers;
use App\Models\BlogPost;
use Illuminate\Http\Request;
class BlogPostController extends Controller
{
public function index()
{
$blogPosts = BlogPost::all();
return response()->json($blogPosts);
}
public function store(Request $request)
{
$blogPost = new BlogPost();
$blogPost->title = $request->input('title');
$blogPost->content = $request->input('content');
$blogPost->save();
return response()->json($blogPost, 201);
}
public function show($id)
{
$blogPost = BlogPost::find($id);
return response()->json($blogPost);
}
public function update(Request $request, $id)
{
$blogPost = BlogPost::find($id);
$blogPost->title = $request->input('title');
$blogPost->content = $request->input('content');
$blogPost->save();
return response()->json($blogPost);
}
public function destroy($id)
{
$blogPost = BlogPost::find($id);
$blogPost->delete();
return response()->json(null, 204);
}
}
This controller defines methods for handling GET, POST, PUT, and DELETE requests to the /blog-posts
endpoint.
Database Migrations and Models
Next, let's create a database migration for our blog post table. Run the following command: php artisan make:migration create_blog_posts_table
.
In the database/migrations
directory, you'll see a new migration file. Open this file and add the following code:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateBlogPostsTable extends Migration
{
public function up()
{
Schema::create('blog_posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->text('content');
$table->timestamps();
});
}
public function down()
{
Schema::dropIfExists('blog_posts');
}
}
This migration defines a new blog_posts
table with id
, title
, content
, and timestamps
columns.
Run the php artisan migrate
command to create the blog_posts
table.
Next, let's create a model for our blog post data. In the app/Models
directory, create a new file called BlogPost.php
. Add the following code:
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class BlogPost extends Model
{
protected $fillable = [
'title',
'content',
];
}
This model defines a simple BlogPost
class that extends the Model
class provided by Eloquent.
Testing the API
Now that we've built our backend API, let's test it using a tool like Postman. Send a GET request to http://localhost:8000/api/blog-posts
to retrieve a list of all blog posts.
Send a POST request to http://localhost:8000/api/blog-posts
with a JSON payload containing the title
and content
fields to create a new blog post.
Send a PUT request to http://localhost:8000/api/blog-posts/1
with a JSON payload containing the updated title
and content
fields to update an existing blog post.
Send a DELETE request to http://localhost:8000/api/blog-posts/1
to delete a blog post.
Conclusion
In this article, we've built a simple backend API using Laravel, covering the basics of controllers, routes, models, and database migrations. We've also tested our API using Postman. Laravel provides a robust set of tools and libraries for building robust and scalable applications, making it an ideal choice for developers of all skill levels. Whether you're building a simple blog or a complex enterprise application, Laravel is a great choice for your next project.
We have a alot more to explore with Laravel like validation middleware services testing security optimizion cache caching security authenticasion middle ware redis testing push notifications amongst other we could discuss