Building a backend with Fastify
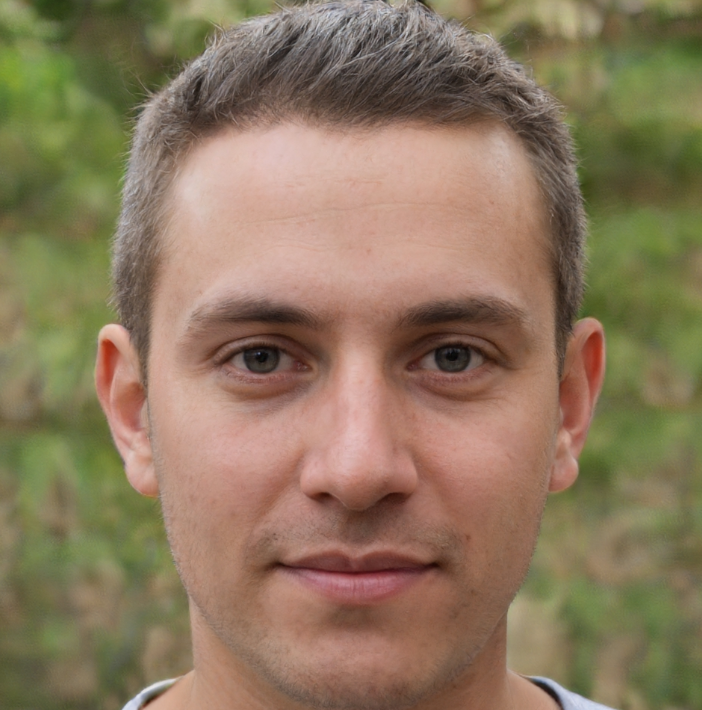

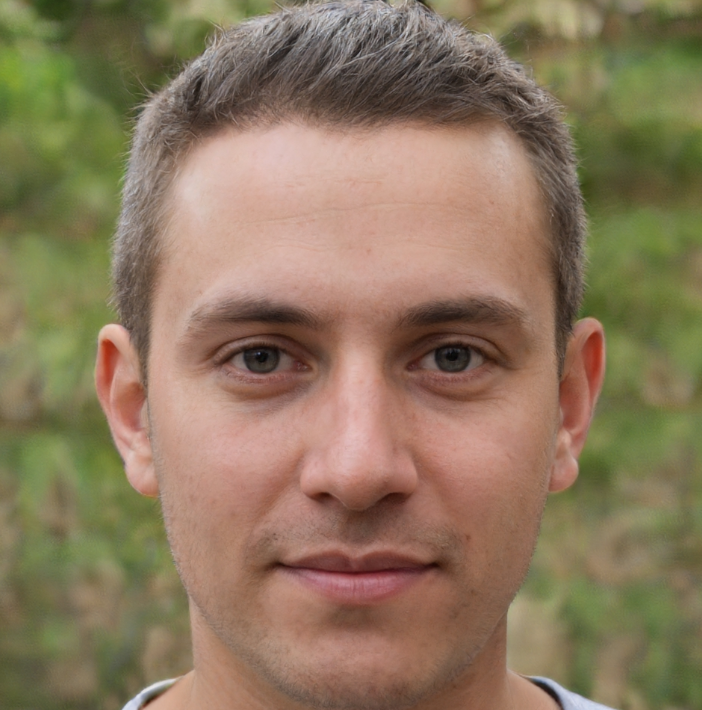
Building a Backend with Fastify
Fastify is a Node.js web framework that's gotten alot of atention lately, and for good reason. It's modern, fast, and low-latency, making it an excellent choice for building robust backend applications. In this article, we're going to explore the process of building a backend with Fastify, covering its core features, setup, and best practices. We'll also look at examples of building a RESTful API and using plugins with Fastify.
What is Fastify?
Fastify is a Node.js web framework that allows developers to build high-performance web applications. It's built on top of the Node.js HTTP module and provides a lightweight and flexible way to handle HTTP requests and responses. Fastify is designed to be fast, scalable, and secure, making it an ideal choice for building modern web applications.
Fastify's core features include:
- High-performance: Fastify is designed to be highly performant and can handle a high volume of requests per second. It's one of the fastest Node.js frameworks out there, and it's perfect for building real-time web applications.
- Low-latency: Fastify has a low-latency response time, making it ideal for applications that require fast response times. This is especially important for applications that require a lot of user interaction, such as games or chat apps.
- Flexible: Fastify allows developers to build custom plugins and extend its functionality. This makes it easy to add new features to your application without having to modify the core framework.
- Secure: Fastify has built-in support for security features such as CORS, CSRF, and SSL/TLS. This makes it easy to secure your application and protect your users' data.
Setting up Fastify
To get started with Fastify, you need to install it using npm or yarn. Here's an example of how to install Fastify using npm:
npm install fastify
Once installed, you can create a new Fastify instance and start building your backend application. Here's an example of a basic Fastify server:
const fastify = require('fastify')();
fastify.get('/', async (request, reply) => {
return { message: 'Hello World!' };
});
fastify.listen(3000, (err, address) => {
if (err) {
console.error(err);
process.exit(1);
}
console.log(`Server listening on ${address}`);
});
This example creates a new Fastify instance and defines a single route for the root URL ('/'). The route returns a JSON response with a message.
Building a RESTful API with Fastify
Fastify is well-suited for building RESTful APIs. Here's an example of how to build a simple RESTful API using Fastify:
const fastify = require('fastify')();
// Define a schema for the API
const schema = {
type: 'object',
properties: {
id: { type: 'integer' },
name: { type: 'string' },
email: { type: 'string' },
},
};
// Define a route for creating a new user
fastify.post('/users', {
schema: {
body: schema,
},
handler: async (request, reply) => {
const user = request.body;
// Save the user to the database
return user;
},
});
// Define a route for retrieving a user by ID
fastify.get('/users/:id', {
schema: {
params: {
type: 'object',
properties: {
id: { type: 'integer' },
},
},
},
handler: async (request, reply) => {
const id = request.params.id;
// Retrieve the user from the database
const user = { id, name: 'John Doe', email: 'john.doe@example.com' };
return user;
},
});
fastify.listen(3000, (err, address) => {
if (err) {
console.error(err);
process.exit(1);
}
console.log(`Server listening on ${address}`);
});
This example defines a schema for the API and two routes: one for creating a new user and one for retrieving a user by ID. The routes use Fastify's built-in support for JSON schema validation to ensure that the request body and parameters conform to the schema.
Using Plugins with Fastify
Fastify has a rich ecosystem of plugins that can be used to extend its functionality. Here's an example of how to use the fastify-mongodb
plugin to connect to a MongoDB database:
const fastify = require('fastify')();
const fastifyMongo = require('fastify-mongodb');
fastify.register(fastifyMongo, {
url: 'mongodb://localhost:27017',
});
fastify.get('/users', {
handler: async (request, reply) => {
const users = await request.mongo.db.collection('users').find().toArray();
return users;
},
});
fastify.listen(3000, (err, address) => {
if (err) {
console.error(err);
process.exit(1);
}
console.log(`Server listening on ${address}`);
});
This example registers the fastify-mongodb
plugin with Fastify and uses it to connect to a MongoDB database. The plugin provides a mongo
property on the request object that can be used to interact with the database.
Best Practices for Building a Backend with Fastify
Here are some best practices for building a backend with Fastify:
- Use a consistant coding style: Fastify uses a consistant coding style throughout its codebase. It's a good idear to follow this style in your own code to ensure consistancy and readability.
- Use plugins to extend functionality: Fastify has a rich ecosystem of plugins that can be used to extend its functionality. Use plugins to add features such as authentication, caching, and database connectivity.
- Use JSON schema validation: Fastify provides built-in support for JSON schema validation. Use this feature to ensure that request bodies and parameters conform to a schema.
- Use async/await: Fastify supports async/await syntax. Use this syntax to write asynchronous code that is easier to read and maintain.
Conclusion
Fastify is a modern, fast, and low-latency web framework for Node.js. It's designed to be highly performant and scalable, making it an excellent choice for building robust backend applications. By following the best practices outlined in this article, you can build a high-performance backend application with Fastify. Remember to use plugins to extend functionality, JSON schema validation to ensure data consistancy, and async/await to write asynchronous code that is easier to read and maintain.