Building a backend with Express.js
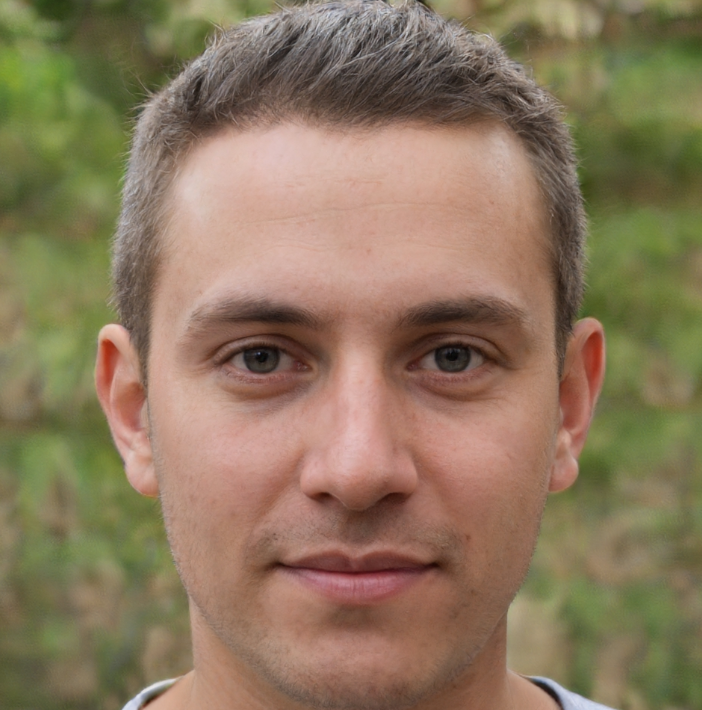

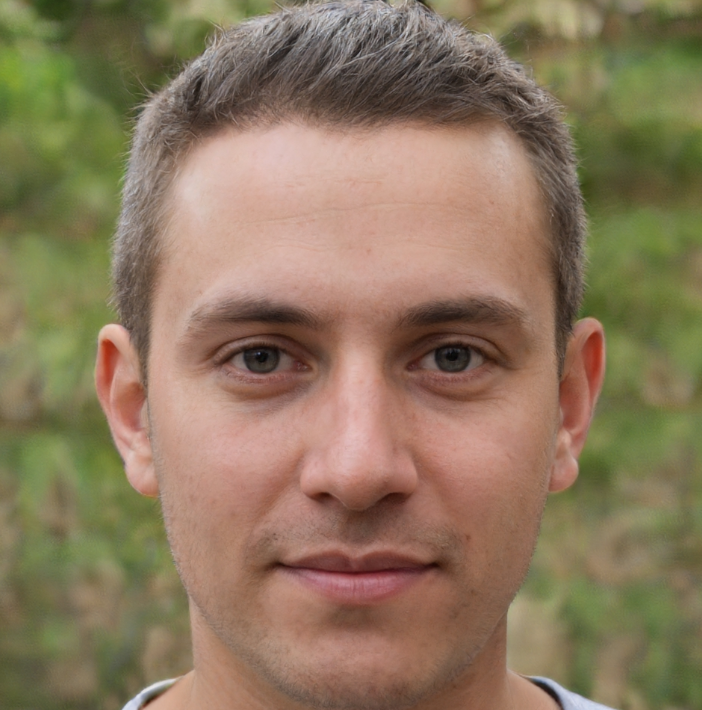
Building a Backend with Express.js: A Comprehensive Guide
Introduction
When it comes to building a scalable and efficient backend for your web application, Express.js is one of the most popular choices among developers. As a flexible and lightweight framework, Express.js provides a robust set of features that make it ideal for building robust APIs and web applications. In this article, we will explore the world of Express.js and provide a comprehensive guide on building a backend using this powerful framework. Wheather your buildig a simple web app or a complext microservices architecture, Express.js is an excelent choice for any Node.js backend project.
Setting up the Project Structure
Before we dive into the world of Express.js, it's essential to set up a proper project structure. This will help us keep our code organized and maintainable throughout the development process. Let's create a new directory for our project and initialize a new Node.js project using the following command:
mkdir myapp
cd myapp
npm init
Next, install Express.js using the following command:
npm install express
Create a new file called app.js
in the root of our project directory. This will be the entry point of our application.
Creating the Express App
In the app.js
file, add the following code to create a new Express app:
const express = require('express');
const app = express();
const port = 3000;
app.listen(port, () => {
console.log(`Server started on port ${port}`);
});
This code creates a new Express app and starts a server on port 3000. We can test the app by running the following command:
node app.js
Open a web browser and navigate to http://localhost:3000
. You should see a blank page, indicating that our server is up and running.
Configuring Middleware
Middleware functions are a crucial part of the Express.js framework. They allow us to perform tasks such as request logging, error handling, and authentication. Let's add a few middleware functions to our app.
Firstly, we'll add a middleware function to log incoming requests:
const logger = (req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
};
app.use(logger);
This middleware function simply logs the incoming request method and URL to the console.
Next, we'll add a middleware function to handle errors:
const errorHandler = (err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
};
app.use(errorHandler);
This middleware function catches any errors that occur during the request-response cycle and logs them to the console. It also sends a generic error message back to the client.
Defining Routes
Routes are the backbone of any web application. They define how our app responds to incoming requests. Let's define a few routes for our app.
Firstly, let's define a route for the index page:
app.get('/', (req, res) => {
res.send('Hello World!');
});
This route responds to GET requests to the root URL and sends a simple "Hello World!" message back to the client.
Next, let's define a route for a fictional API endpoint:
app.get('/api/users', (req, res) => {
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Doe' },
];
res.json(users);
});
This route responds to GET requests to the /api/users
endpoint and sends a JSON response containing a list of users.
Working with Databases
In any real-world application, we'll need to interact with a database to store and retrieve data. Let's use the popular MongoDB database for our app.
Firstly, install the mongodb
package using the following command:
npm install mongodb
Next, create a new file called db.js
in the root of our project directory. This file will contain our database configuration:
const MongoClient = require('mongodb').MongoClient;
const url = 'mongodb://localhost:27017';
const dbName = 'myapp';
const client = new MongoClient(url);
const db = client.db(dbName);
module.exports = db;
In this file, we create a new MongoDB client and connect to the myapp
database.
Finally, let's update our /api/users
endpoint to retrieve data from the database:
const db = require('./db');
app.get('/api/users', (req, res) => {
db.collection('users').find().toArray((err, users) => {
if (err) {
res.status(500).send(err);
} else {
res.json(users);
}
});
});
In this updated endpoint, we use the db
object to retrieve a list of users from the users
collection and send it back to the client.
Error Handling and Logging
Error handling and logging are crucial components of any backend application. Express.js provides a built-in error-handling mechanism, but we can also use third-party libraries like Winston for logging.
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.Console(),
new winston.transports.File({ filename: 'error.log', level: 'error' })
]
});
app.use((err, req, res, next) => {
logger.error(err);
res.status(500).send({ message: 'Internal Server Error' });
});
In this example, we're creating a new logger instance with Winston and specifying a log level, format, and transports. We're then using this logger to handle errors in our application.
Conclusion
In this comprehensive guide, we've explored the world of Express.js and built a robust backend for our web application. We've set up a project structure, created an Express app, configured middleware, defined routes, worked with a database, and handled errors. With this knowledge, you're ready to build your own scalable and efficient backend using Express.js. Remember to keep practicing and experimenting with different features and techniques to become a master of Express.js.