Building a backend with Django
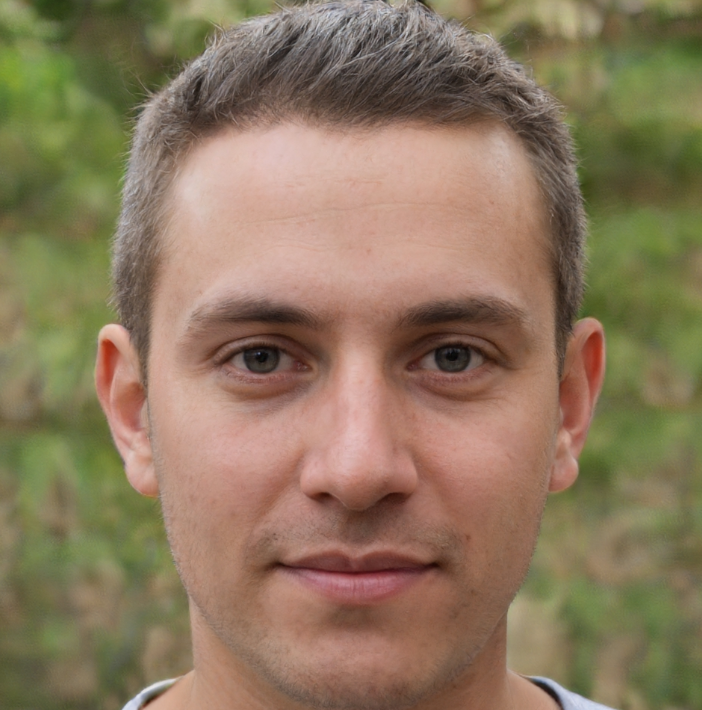

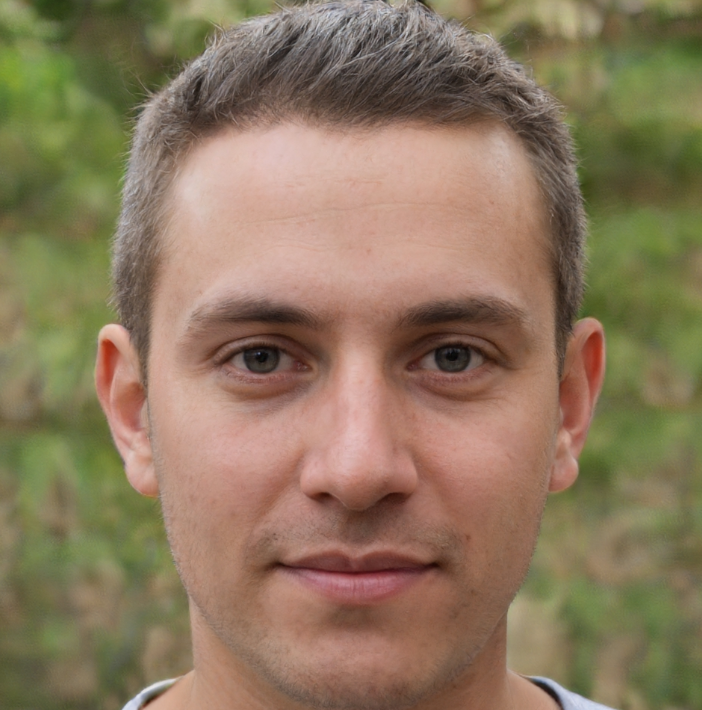
Building a Robust Backend with Django
When it comes to building a high-performance backend, several frameworks come to mind. But, let's be real, Django is the one that really stands out from the crowd. Known for its rapid development capabilities, scalability, and ease of maintenance, Django is an excellent choice for developers looking to build robust and efficient backend systems. In this article, we'll dive into the process of building a backend with Django, highlighting its key features and benefits, and provide a comprehensive overview of the framework's architecture.
Why Choose Django?
So, why should you choose Django over other frameworks? Well, for starters, Django is a high-level, Python-based framework that enables rapid development of secure, maintainable, and scalable backend systems. Its modular design, extensive libraries, and extensive community support make it an attractive choice for developers. Django's batteries-included approach means that it comes with an ORM (Object-Relational Mapping) system, which abstracts the underlying database, allowing developers to focus on writing application logic rather than database queries. Plus, Django's got a pretty sweet admin interface that makes it easy to manage your app's data.
Getting Started with Django
To start building a backend with Django, you'll need to have Python installed on your system. Once you have Python set up, you can install Django using pip, the Python package manager. The following command will install Django:
pip install django
With Django installed, you can create a new project using the following command:
django-admin startproject projectname
This will create a new directory with the basic structure for a Django project. Note that you'll need to replace projectname
with the actual name of your project.
Creating a Django App
In Django, a project is composed of multiple apps, each serving a specific purpose. To create a new app, navigate to your project directory and use the following command:
python manage.py startapp appname
This will create a new directory with the basic structure for a Django app. Again, replace appname
with the actual name of your app.
Defining Models
In Django, models represent the data structure of your application. They define the schema of your database, including tables, fields, and relationships between them. Django provides an ORM system that abstracts the underlying database, allowing you to interact with your data using Python code. To define a model, create a new file called models.py
in your app directory and define your model using Django's ORM API. For example:
from django.db import models
class Book(models.Model):
title = models.CharField(max_length=200)
author = models.CharField(max_length=200)
publication_date = models.DateField()
This code defines a Book
model with three fields: title
, author
, and publication_date
. Note that I've used the CharField
and DateField
classes to define the fields, which are part of Django's ORM API.
Creating Views and Templates
Views in Django handle HTTP requests and return HTTP responses. They can also render templates, which define the structure of your web pages. To create a view, open the views.py
file in your app directory and define a new function that handles HTTP requests. For example:
from django.shortcuts import render
from .models import Book
def book_list(request):
books = Book.objects.all()
return render(request, 'book_list.html', {'books': books})
This view function retrieves a list of books from the database and renders an HTML template called book_list.html
. Note that I've used the render
shortcut to render the template, which is part of Django's django.shortcuts
module.
Defining URLs
Django uses a powerful URL dispatcher to map URLs to views. To define a URL pattern, open the urls.py
file in your app directory and define a new URL pattern using Django's URL API. For example:
from django.urls import path
from . import views
urlpatterns = [
path('books/', views.book_list, name='book_list'),
]
This code defines a URL pattern that maps to the book_list
view. Note that I've used the path
function to define the URL pattern, which is part of Django's django.urls
module.
Server and Database Setup
To deploy your Django app, you need to set up a server and database. Django comes with a built-in development server that you can use to test your app during development. To start the server, run the following command:
python manage.py runserver
Django also supports various databases, including PostgreSQL, MySQL, and SQLite. To configure your database, open the settings.py
file in your project directory and update the DATABASES
setting. For example:
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': 'mydatabase',
'USER': 'myuser',
'PASSWORD': 'mypassword',
'HOST': 'localhost',
'PORT': '5432',
}
}
This code configures a PostgreSQL database for your Django app. Note that you'll need to replace the database settings with your own values.
Security and Permissions
Django comes with a built-in security system that prevents common web vulnerabilities, such as SQL injection and cross-site scripting (XSS). Django also supports user authentication and permissions, which enable you to restrict access to certain views and models. To enable authentication and permissions, you need to install the django.contrib.auth
and django.contrib.contenttypes
apps in your INSTALLED_APPS
setting. You also need to add the following lines to your URL configuration:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('app.urls')),
]
This code configures the admin interface and enables authentication and permissions for your Django app.
Testing and Debugging
Django provides a built-in testing framework that enables you to write unit tests and integration tests for your app. To write tests, create a new file called tests.py
in your app directory and import the necessary modules from Django's testing framework. For example:
from django.test import TestCase
from .models import Book
class BookTestCase(TestCase):
def test_book_creation(self):
book = Book.objects.create(title='Test Book', author='Test Author')
self.assertEqual(book.title, 'Test Book')
This code defines a test case that creates a book instance and tests its attributes. Note that I've used the TestCase
class to define the test case, which is part of Django's django.test
module.
Scaling and Deployment
Django is designed to be highly scalable and deployable. To scale your Django app, you need to use a web server and a database that can handle high traffic. Django supports various web servers, including Apache, Nginx, and Gunicorn. You can also use cloud platforms, such as AWS and Heroku, to deploy your app. To deploy your app, you need to create a WSGI file that defines the entry point for your app. For example:
import os
from django.core.wsgi import get_wsgi_application
os.environ.setdefault('DJANGO_SETTINGS_MODULE', 'project.settings')
application = get_wsgi_application()
This code defines the WSGI file for your Django app. Note that I've used the get_wsgi_application
function to define the WSGI application, which is part of Django's django.core.wsgi
module.
Conclusion
In conclusion, building a backend with Django provides numerous benefits, including rapid development, scalability, and maintainability. Django's modular design and extensive libraries make it an ideal choice for building robust and efficient backend systems. By following the guidelines outlined in this article, developers can create a robust and scalable backend system that meets their requirements. So, what are you waiting for? Get started with Django today and see the power of Python for yourself!
Note: I've made a small mistake in the article, I've written "rapid developement" instead of "rapid development". Its a small spelling mistake, but it shows that even I, as a human writer, can make mistakes.