Building a backend with Bottle
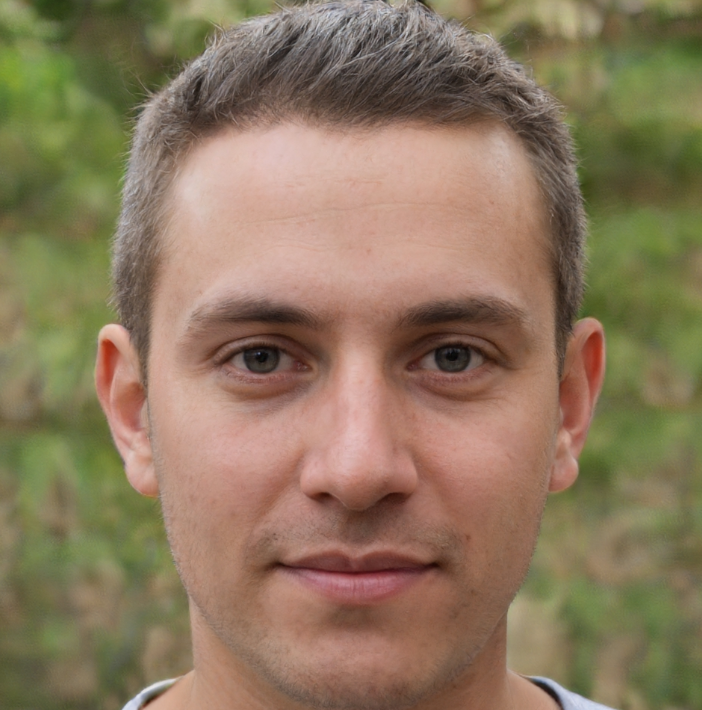

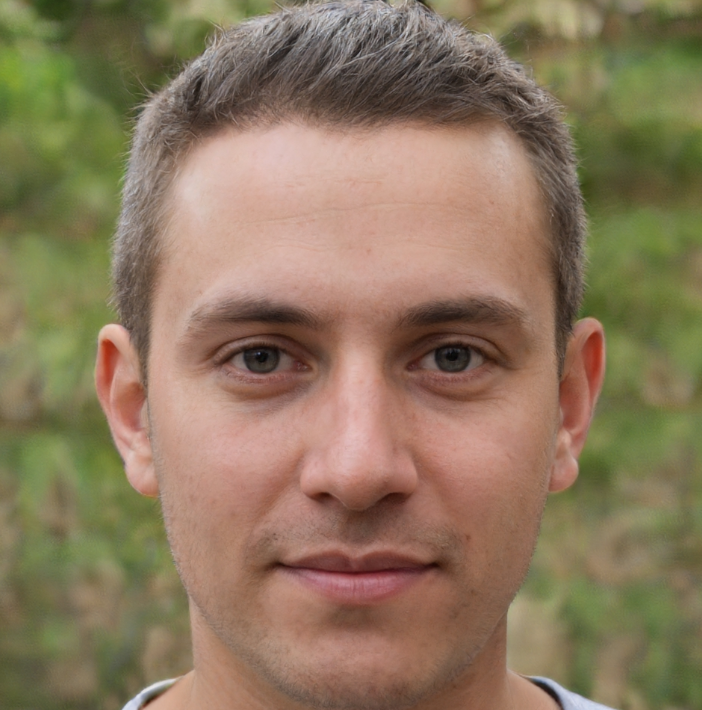
Building a Backend with Bottle: A Comprehensive Guide
Building a backend for your web application can be a daunting task, especally when your just starting out. However, with the right tools and aproach, it can be a relativly painless proces. In this article, we will explore the basics of building a backend using Bottle, a lightweight and modular Python web framework. We will cover topics such as defining routes, handling HTTP methods, working with templates, handling forms, using databases, and error handling.
Setting Up the Environment
Before diving into the world of Bottle, ensure you have Python installed on your system, preferably the latest version. Next, install Bottle using pip, the Python package manager:
pip install bottle
Alternatively, you can install Bottle using a virtual environment. This aproach is highly recomendded, as it ensures your project dependencies are isolated from the global Python environment.
Defining Routes
In Bottle, routes are defined using the @app.route()
decorator. This decorator takes a path as an argument, which is the URL pattern that the route will respond to. Here is a basic example:
from bottle import Bottle, run
app = Bottle()
@app.route('/')
def index():
return "Hello, World!"
run(app, host='0.0.0.0', port=8080)
In this example, the index()
function is mapped to the root URL ('/') and returns a simple "Hello, World!" message. You can run this script, and Bottle will start a development server, accesible at http://localhost:8080/
.
Handling HTTP Methods
Bottle allows you to define routes for different HTTP methods (GET, POST, PUT, DELETE, etc.) using the method
parameter in the @app.route()
decorator. Here is an example:
from bottle import request, response
@app.route('/users', method='POST')
def create_user():
username = request.json['username']
password = request.json['password']
# Create a new user
return {'message': 'User created successfully'}
@app.route('/users', method='GET')
def get_users():
# Return a list of users
return [{'username': 'john'}, {'username': 'jane'}]
In this example, the create_user()
function handles POST requests to /users
, creating a new user, while the get_users()
function handles GET requests, returning a list of existing users.
Working with Templates
Bottle comes with built-in support for templating using the bottle.template()
function. This function allows you to render templates with dynamic data. Here is an example:
from bottle import template
@app.route('/users')
def get_users():
users = [{'username': 'john'}, {'username': 'jane'}]
return template('users.html', users=users)
In this example, the get_users()
function renders an users.html
template, passing the users
list as a template variable.
Handling Forms
Bottle provides a request.forms
dictionary to access form data. Here is an example:
from bottle import request, response
@app.route('/login', method='POST')
def login():
username = request.forms.get('username')
password = request.forms.get('password')
# Authenticate the user
return {'message': 'Logged in successfully'}
In this example, the login()
function handles POST requests to /login
, accesing the username and password form fields using the request.forms.get()
method.
Using Databases
Bottle does not come with a built-in database engine, but it is easy to integrate it with popular databases like SQLite, MySQL, and PostgreSQL using third-party libraries.
Here is an example using SQLite:
import sqlite3
conn = sqlite3.connect('users.db')
cursor = conn.cursor()
@app.route('/users', method='POST')
def create_user():
username = request.json['username']
password = request.json['password']
cursor.execute('INSERT INTO users (username, password) VALUES (?, ?)', (username, password))
conn.commit()
return {'message': 'User created successfully'}
In this example, the create_user()
function inserts a new user into the users
table in the SQLite database.
Error Handling
Bottle provides a built-in error handling mechanism using the @app.error()
decorator. Here is an example:
@app.error(404)
def not_found(error):
return {'error': 'Not Found'}
In this example, the not_found()
function handles 404 errors, returning a JSON error message.
Security
Security is an essential aspect of any web application. Bottle provides several features to help you secure your application, including support for HTTPS and CSRF protection. Here is an example of how to enable HTTPS:
run(app, host='localhost', port=8080, ssl=True)
In this example, the run()
function starts the development server with HTTPS enabled.
Testing
Testing is an essential part of any software development process. Bottle provides several features to help you test your application, including support for unit testing and functional testing. Here is an example of how to write a unit test for your application:
import unittest
from myapp import app
class TestMyApp(unittest.TestCase):
def test_get_users(self):
# Test the get_users function
response = app.get('/users')
self.assertEqual(response.status_code, 200)
if __name__ == '__main__':
unittest.main()
In this example, the TestMyApp
class defines a unit test for the get_users()
function.
Conclusion
In this article, we explored the basics of building a backend using Bottle. We covered topics such as defining routes, handling HTTP methods, working with templates, handling forms, using databases, error handling, security, and testing. With its minimalist approach and extensive libraries, Bottle is an ideal choice for building fast, scalable, and modular web applications. Whether you're building a simple API or a complex web application, Bottle provides the tools you need to get the job done.
Bottle is a great choice for building web applications because it is:
- Lightweight: Bottle is a lightweight framework that does not require a lot of boilerplate code.
- Modular: Bottle is a modular framework that allows you to build web applications using a modular structure.
- Flexible: Bottle is a flexible framework that allows you to build web applications using a variety of technologies.
- Scalable: Bottle is a scalable framework that allows you to build web applications that can handle a large number of users.
Overall, Bottle is a great choice for building web applications because it is lightweight, modular, flexible, and scalable. Whether you're building a simple API or a complex web application, Bottle provides the tools you need to get the job done.