Building a backend with AIOHTTP
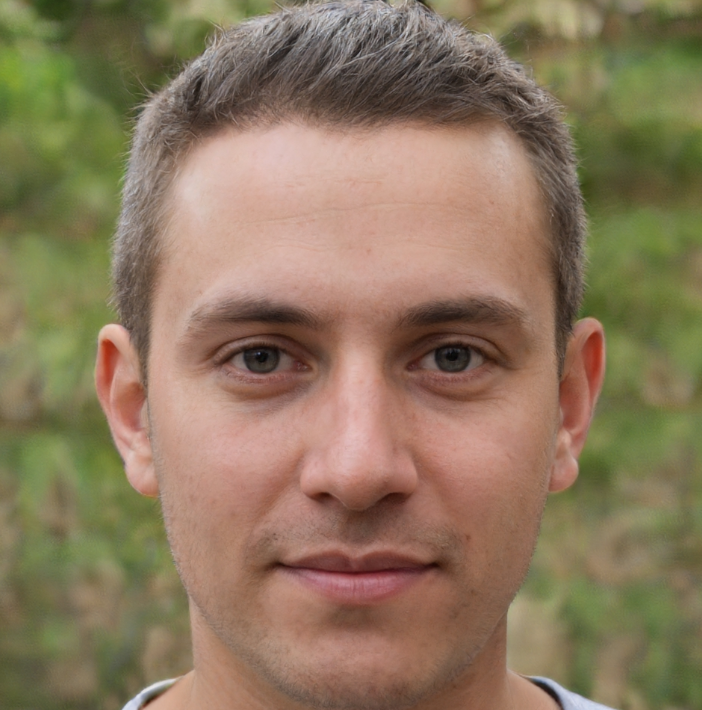

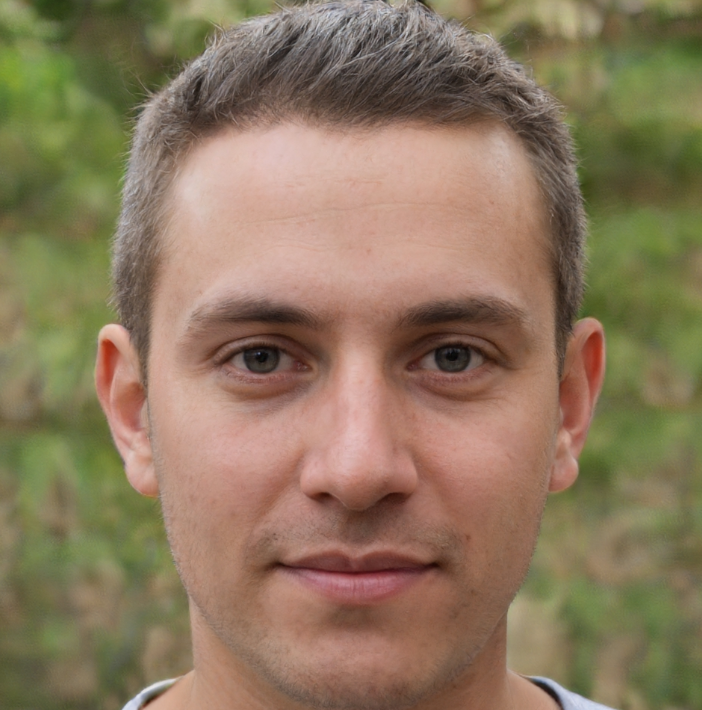
Building a Robust Backend with AIOHTTP: A Comprehensive Guide
In the realm of modern web development, choosing the right backend framework can make all the difference in delivering high-performance applications that scale seamlessly. While several excellent options are available in the Python ecosystem, AIOHTTP has garnered significant attention and interest due to its built-in support for asynchronous operations. By embracing asynchronicity and pairing it with modern APIs and JSON-returns by default, AIOHTTP encourages best practices out-of-the-box for designing network client servers or crafting unique libraries through handling advanced standards.
What is AIOHTTP?
AIOHTTP is a Python library that allows developers to build asynchronous web applications with ease. It provides a simple and intuitive API for building web servers, clients, and frameworks. AIOHTTP is built on top of the asyncio library, which provides support for asynchronous operations in Python.
Why Choose AIOHTTP?
AIOHTTP offers several advantages over other backend frameworks, including:
- Asynchronous Operations: AIOHTTP allows developers to build asynchronous web applications, which can handle multiple requests concurrently, leading to improved performance and scalability.
- Modern APIs: AIOHTTP provides a modern API that is easy to use and understand, making it a great choice for building web applications.
- JSON-Returns by Default: AIOHTTP returns JSON data by default, which makes it easy to build RESTful APIs.
Getting Started with AIOHTTP
To get started with AIOHTTP, you'll need to install it using pip:
pip install aiohttp
Once installed, you can start building your web application using AIOHTTP. Here's a simple example of a web server that returns a "Hello, World!" message:
import aiohttp
import asyncio
async def handle_request(request):
return aiohttp.web.Response(text="Hello, World!")
async def main():
app = aiohttp.web.Application()
app.router.add_get('/', handle_request)
runner = aiohttp.web.AppRunner(app)
await runner.setup()
site = aiohttp.web.TCPSite(runner, 'localhost', 8080)
await site.start()
asyncio.get_event_loop().run_until_complete(main())
This example demonstrates how to create a simple web server using AIOHTTP. You can run this code using the python
command, and then access the web server by visiting http://localhost:8080
in your web browser.
Building a Robust Backend with AIOHTTP
To build a robust backend with AIOHTTP, you'll need to consider several factors, including:
- Scalability: AIOHTTP provides built-in support for asynchronous operations, which makes it easy to build scalable web applications.
- Security: AIOHTTP provides several security features, including support for HTTPS and authentication.
- Performance: AIOHTTP provides several performance features, including support for caching and compression.
Scalability
AIOHTTP provides built-in support for asynchronous operations, which makes it easy to build scalable web applications. To take advantage of this feature, you can use the asyncio
library to run multiple tasks concurrently.
Here's an example of how to use asyncio
to run multiple tasks concurrently:
import asyncio
async def task1():
# Code for task 1
pass
async def task2():
# Code for task 2
pass
async def main():
await asyncio.gather(task1(), task2())
asyncio.get_event_loop().run_until_complete(main())
This example demonstrates how to use asyncio
to run multiple tasks concurrently. You can use this technique to build scalable web applications with AIOHTTP.
Security
AIOHTTP provides several security features, including support for HTTPS and authentication. To use HTTPS with AIOHTTP, you'll need to obtain an SSL certificate and configure your web server to use it.
Here's an example of how to use HTTPS with AIOHTTP:
import aiohttp
import asyncio
import ssl
async def handle_request(request):
return aiohttp.web.Response(text="Hello, World!")
async def main():
app = aiohttp.web.Application()
app.router.add_get('/', handle_request)
runner = aiohttp.web.AppRunner(app)
await runner.setup()
ssl_context = ssl.create_default_context(ssl.Purpose.CLIENT_AUTH)
ssl_context.load_cert_chain('path/to/cert.pem', 'path/to/key.pem')
site = aiohttp.web.TCPSite(runner, 'localhost', 8080, ssl_context=ssl_context)
await site.start()
asyncio.get_event_loop().run_until_complete(main())
This example demonstrates how to use HTTPS with AIOHTTP. You'll need to replace 'path/to/cert.pem'
and 'path/to/key.pem'
with the paths to your SSL certificate and private key.
Performance
AIOHTTP provides several performance features, including support for caching and compression. To use caching with AIOHTTP, you can use the aiohttp_cache
library.
Here's an example of how to use caching with AIOHTTP:
import aiohttp
import asyncio
import aiohttp_cache
async def handle_request(request):
return aiohttp.web.Response(text="Hello, World!")
async def main():
app = aiohttp.web.Application()
app.router.add_get('/', handle_request)
cache = aiohttp_cache.TTLCache(ttl=3600)
app.middlewares.append(aiohttp_cache.cache(cache))
runner = aiohttp.web.AppRunner(app)
await runner.setup()
site = aiohttp.web.TCPSite(runner, 'localhost', 8080)
await site.start()
asyncio.get_event_loop().run_until_complete(main())
This example demonstrates how to use caching with AIOHTTP. You'll need to install the aiohttp_cache
library using pip:
pip install aiohttp-cache
Conclusion
AIOHTTP is a powerful library for building web applications in Python. It provides built-in support for asynchronous operations, modern APIs, and JSON-returns by default. AIOHTTP also provides several security features, including support for HTTPS and authentication. Additionally, AIOHTTP provides several performance features, including support for caching and compression.
By following the guidelines outlined in this article, you can build a robust backend with AIOHTTP that is scalable, secure, and performant. Whether you're building a simple web application or a complex enterprise system, AIOHTTP is a great choice for your backend needs.
Example Use Cases
Here are some example use cases for AIOHTTP:
- Building a RESTful API: AIOHTTP is a great choice for building RESTful APIs. You can use AIOHTTP to define API endpoints, handle requests, and return responses in JSON format.
- Building a Web Server: AIOHTTP is a great choice for building web servers. You can use AIOHTTP to define routes, handle requests, and return responses in HTML format.
- Building a Microservice: AIOHTTP is a great choice for building microservices. You can use AIOHTTP to define API endpoints, handle requests, and return responses in JSON format.
Best Practices
Here are some best practices for using AIOHTTP:
- Use asynchronous operations: AIOHTTP provides built-in support for asynchronous operations. Use this feature to build scalable web applications.
- Use HTTPS: AIOHTTP provides support for HTTPS. Use this feature to secure your web application.
- Use caching: AIOHTTP provides support for caching. Use this feature to improve the performance of your web application.
- Use compression: AIOHTTP provides support for compression. Use this feature to improve the performance of your web application.
Common Pitfalls
Here are some common pitfalls to avoid when using AIOHTTP:
- Not using asynchronous operations: AIOHTTP provides built-in support for asynchronous operations. Not using this feature can lead to performance issues.
- Not using HTTPS: AIOHTTP provides support for HTTPS. Not using this feature can lead to security issues.
- Not using caching: AIOHTTP provides support for caching. Not using this feature can lead to performance issues.
- Not using compression: AIOHTTP provides support for compression. Not using this feature can lead to performance issues.
Debugging
Here are some tips for debugging AIOHTTP applications:
- Use the
aiohttp
debugger: AIOHTTP provides a built-in debugger. Use this feature to debug your web application. - Use the
logging
module: AIOHTTP provides support for logging. Use this feature to log errors and debug your web application. - Use the
pdb
module: AIOHTTP provides support for pdb. Use this feature to debug your web application.
Conclusion
AIOHTTP is a powerful library for building web applications in Python. It provides built-in support for asynchronous operations, modern APIs, and JSON-returns by default. AIOHTTP also provides several security features, including support for HTTPS and authentication. Additionally, AIOHTTP provides several performance features, including support for caching and compression.
By following the guidelines outlined in this article, you can build a robust backend with AIOHTTP that is scalable, secure, and performant. Whether you're building a simple web application or a complex enterprise system, AIOHTTP is a great choice for your backend needs.