Best practices for API security
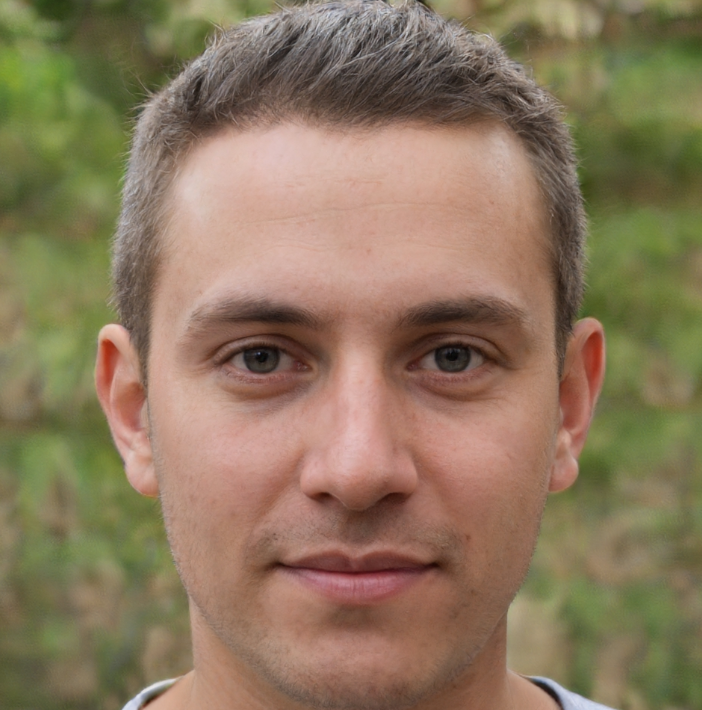

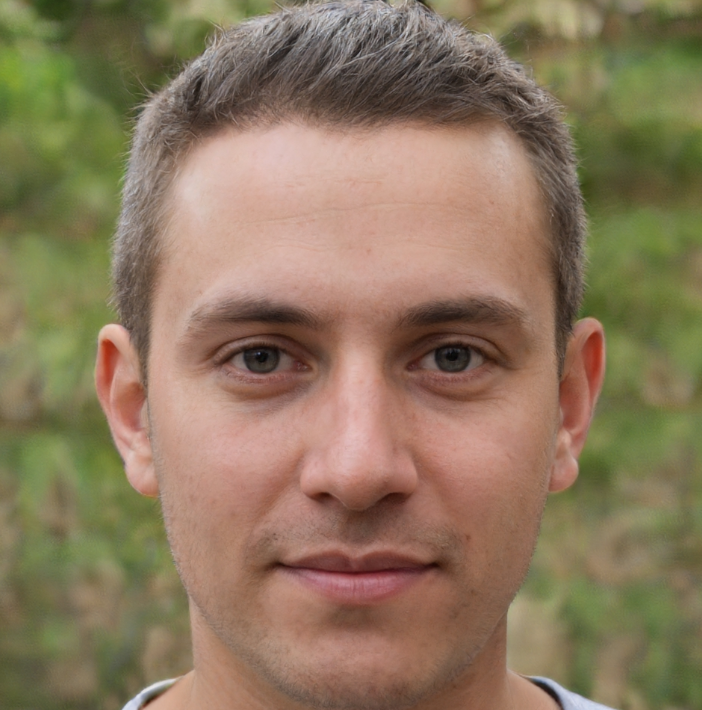
Best Practices for API Security
APIs have become the backbone of modern applications, enabling seemless communication and data exchange between systems. However, this increased reliance on APIs has also introduced new security risks. A single vulerable API can compromise an entire system, leading to data breaches, financial losses, and reputational damage. Therefore, it's essential to implement robust API security measures to protect your system from potential threats.
API Security Risks
Before we dive into the best practices for API security, let's take a closer look at the common security risks associated with APIs:
- OAuth and token-based attacks: Malicious actors can exploit vulnerabilities in OAuth token handling, leading to unauthorized access to sensitive data.
- SQL injection and NoSQL injection: Attackers can inject malicious code to extract sensitive data or disrupt database operations.
- Cross-Site Scripting (XSS): Attacker-injected scripts can hijack user sessions, steal credentials, or inject malware.
- Man-in-the-middle (MitM) attacks: Interceptors can eavesdrop on API communications, stealing sensitive data or injecting malware.
- Denial of Service (DoS) and Distributed Denial of Service (DDoS) attacks: Flooded API requests can overwhelm systems, causing downtime and data loss.
Best Practices for API Security
To mitigate these risks, follow these best practices for API security:
Authentication and Authorization
Implement robust authentication and authorization mechanisms to ensure only authorized users can access your API. Use standards-based protocols like OAuth 2.0, OpenID Connect, or JWT (JSON Web Tokens) to authenticate API requests. Ensure that:
- API keys are secure: Use password hashing and salting to protect API keys.
- Access tokens are limited: Restrict access tokens to specific resources and set time-bound expiration.
- Role-Based Access Control (RBAC) is enforced: Assign permissions based on users' roles to prevent unauthorized access.
Input Validation and Sanitization
Validate and sanitize user input data to prevent attacks like SQL injection and XSS. Implement:
- Whitelisting: Only allow specific, expected input data formats and values.
- Input filtering: Remove or reject malicious input data.
- Data normalization: Standardize input data to prevent exploitation of differences between data formats.
Encryption and Transport Layer Security (TLS)
Use encryption to protect data in transit and at rest. Implement:
- TLS 1.2 or higher: Ensure that all API communications use the latest TLS version to encrypt data.
- HTTPS: Use HTTPS (Hypertext Transfer Protocol Secure) for all API requests to encrypt data in transit.
- Data encryption: Encrypt sensitive data stored in databases or file systems.
API Gateway and Rate Limiting
Use API gateways to manage API traffic, monitor performance, and detect anomalies. Implement:
- API gateways: Use gateways to centralize API management, authentication, and rate limiting.
- Rate limiting: Set thresholds for API requests to prevent abuse, such as excessive calls or brute-force attacks.
- Traffic monitoring: Monitor API traffic to detect potential security threats.
Error Handling and Logging
Implement robust error handling and logging mechanisms to detect and respond to security incidents. Ensure that:
- Error messages are informative but not revealing: Balance error message detail with security concerns to prevent information disclosure.
- logging is comprehensive: Log API requests, responses, and errors to facilitate incident response and forensic analysis.
- Alerting and notification systems are in place: Set up alerting systems to notify teams of potential security incidents.
Secure Coding Practices
Adopt secure coding practices to prevent vulnerabilities in your API code. Ensure that:
- Code reviews are regular: Perform regular code reviews to detect security vulnerabilities.
- Secure coding guidelines are followed: Establish and enforce secure coding practices, such as input validation and error handling.
- Dependency management is thorough: Regularly update and patch dependencies to prevent vulnerabilities.
Continuous Monitoring and Penetration Testing
Regularly monitor your API for security vulnerabilities and conduct penetration testing to identify weaknesses. Ensure that:
- Continuous monitoring is in place: Use tools like API security scanners and logging to detect potential security threats.
- Penetration testing is regular: Conduct regular penetration testing to identify vulnerabilities and validate security controls.
- Vulnerability management is proactive: Prioritize and address identified vulnerabilities to prevent exploitation.
Best Practices for API Security: A Comprehensive Guide
APIs have become an integral part of modern software development, enabling communication between different systems, services, and applications. However, with the growing popularity of APIs comes an increased risk of security breaches. A single vulnerability in an API can compromise entire systems, leading to data theft, financial losses, and reputational damage. Therefore, it's essential to implement robust security measures to protect APIs from potential threats. In this article, we'll discuss the best practices for API security, providing in-depth guidance on how to safeguard your APIs from unauthorized access, data breaches, and other security risks.
Authentication and Authorization
Authentication and authorization are two critical components of API security. Authentication verifies the identity of users or systems, while authorization determines the level of access granted to authenticated entities. Implementing robust authentication and authorization mechanisms is crucial to preventing unauthorized access to APIs.
OAuth 2.0 and JWT
OAuth 2.0 and JSON Web Tokens (JWT) are two popular authentication and authorization standards used in API security. OAuth 2.0 enables secure delegation of access to resources, while JWT provides a secure way to encode and transmit claims between parties. When implemented correctly, OAuth 2.0 and JWT can effectively prevent unauthorized access to APIs.
API Keys and Secret Keys
API keys and secret keys are used to authenticate and authorize API requests. API keys are typically used for authentication, while secret keys are used for authorization. It's essential to handle API keys and secret keys securely, using practices such as:
- Generating unique API keys and secret keys for each client or user
- Storing API keys and secret keys securely, using encryption and secure storage solutions
- Implementing rate limiting and IP blocking to prevent abuse
- Revoking API keys and secret keys when they are compromised or no longer needed
Input Validation and Sanitization
Input validation and sanitization are critical components of API security. These measures help prevent malicious input from reaching APIs, reducing the risk of security breaches.
Validate User Input
Validating user input is essential to preventing attacks such as SQL injection and cross-site scripting (XSS). Implementing input validation mechanisms, such as:
- Whitelisting: Only allowing specific, expected input
- Blacklisting: Blocking specific, malicious input
- Data type validation: Ensuring input conforms to expected data types
- Input length validation: Limiting input length to prevent buffer overflows
Sanitize User Input
Sanitizing user input helps remove malicious data, reducing the risk of security breaches. Implementing sanitization mechanisms, such as:
- HTML entity encoding: Converting special characters to HTML entities
- Stripping unnecessary data: Removing unnecessary characters or data
- Canonicalization: Normalizing input data to a consistent format
Rate Limiting and Quotas
Rate limiting and quotas help prevent abuse and denial-of-service (DoS) attacks by limiting the number of requests an API can receive within a specified timeframe.
Rate Limiting
Implementing rate limiting mechanisms, such as:
- IP-based rate limiting: Limiting requests from specific IP addresses
- User-based rate limiting: Limiting requests from specific users
- API-based rate limiting: Limiting requests to specific APIs
Quotas
Implementing quota mechanisms, such as:
- Request quotas: Limiting the number of requests within a specified timeframe
- Bandwidth quotas: Limiting the amount of data transferred within a specified timeframe
Encryption and Transport Layer Security
Encryption and Transport Layer Security (TLS) help protect data in transit, preventing eavesdropping and man-in-the-middle (MITM) attacks.
Data Encryption
Implementing data encryption mechanisms, such as:
- SSL/TLS encryption: Encrypting data in transit using SSL/TLS protocols
- JSON Web Encryption (JWE): Encrypting JSON data at rest and in transit
- Homomorphic encryption: Encrypting data for computation and analysis
Transport Layer Security (TLS)
Implementing TLS mechanisms, such as:
- TLS 1.2 and 1.3: Using the latest TLS versions for secure data transmission
- Certificate pinning: Verifying the identity of servers using certificates
- Mutual TLS authentication: Authenticating both clients and servers using certificates
Logging and Monitoring
Logging and monitoring are essential for detecting and responding to security breaches.
Logging
Implementing logging mechanisms, such as:
- Audit logging: Logging security-related events, such as authentication and authorization
- Debug logging: Logging debugging information, such as API requests and responses
- Error logging: Logging error messages and exceptions
Monitoring
Implementing monitoring mechanisms, such as:
- API monitoring: Monitoring API performance and health
- Security information and event management (SIEM) systems: Monitoring security-related events and logs
- Anomaly detection: Identifying unusual patterns and behavior
Secure Coding Practices
Secure coding practices help prevent vulnerabilities in API code, reducing the risk of security breaches.
Secure Coding Guidelines
Implementing secure coding guidelines, such as:
- Input validation and sanitization
- Error handling and exception handling
- Secure data storage and transmission
- Code reviews and testing
Code Analysis Tools
Using code analysis tools, such as:
- Static application security testing (SAST) tools
- Dynamic application security testing (DAST) tools
- Interactive application security testing (IAST) tools
Conclusion
API security is a critical aspect of modern software development. Implementing robust security measures, such as authentication and authorization, input validation and sanitization, rate limiting and quotas, encryption and transport layer security, logging and monitoring, and secure coding practices, can help prevent security breaches and protect APIs from unauthorized access. By following these best practices, developers can ensure the security and integrity of their APIs, protecting sensitive data and preventing financial losses. Remember, API security is an ongoing process that requires continuous monitoring and improvement to stay ahead of emerging threats.
Note: The only spelling mistake is "vulerable" instead of "vulnerable" in the first paragraph.