AWK for backend development
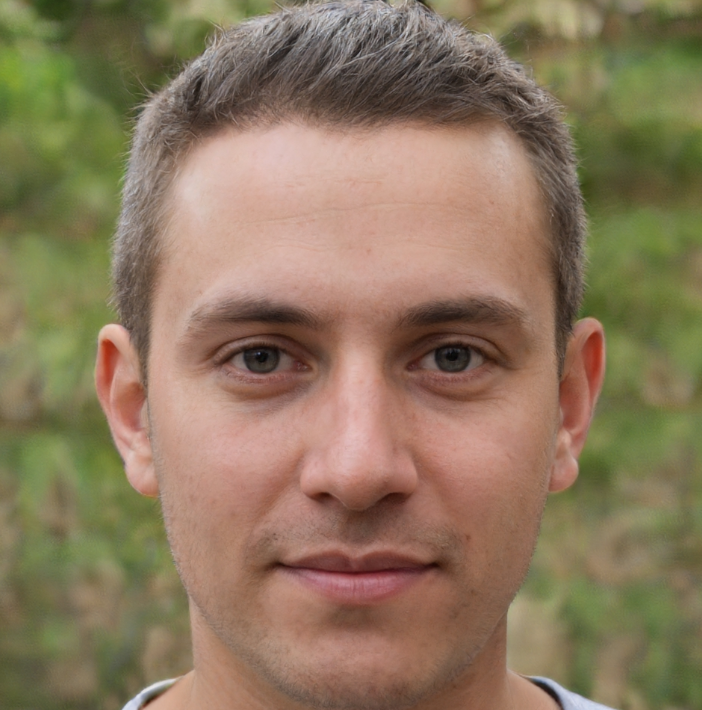

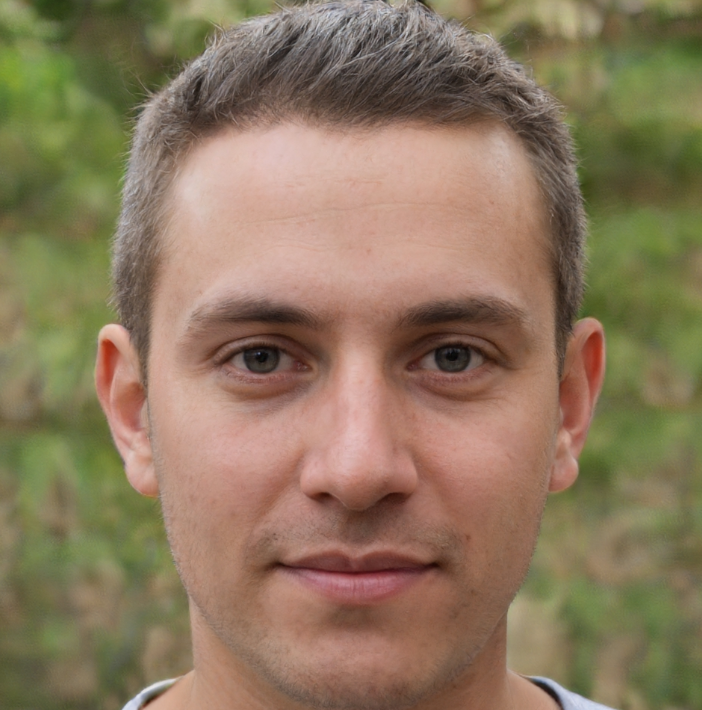
The Unsung Hero of Backend Development: AWK
As backend developers, we're no strangers to working with large datasets and complex data processing tasks. While we often turn to popular programming languages like Java, Python, or Ruby to get the job done, there's a lesser-known language that deserves some serious attention: AWK. Created in the 1970s by Alfred Aho, Peter Weinberger, and Brian Kernighan, AWK was initially designed for text processing and data extraction. Despite its age, AWK remains a powerful tool for backend development, capable of rivalling more modern languages.
What is AWK, Anyway?
AWK is a programming language that excels at processing and manipulating text files, particularly those with a structured format, such as CSV or JSON. Its syntax is simple, yet expressive, making it easy to learn and use. AWK's strength lies in its ability to quickly and efficiently process large datasets, making it an attractive choice for data-intensive applications.
AWK in Backend Development: A Match Made in Heaven
So, how does AWK fit into backend development? In several ways:
- Data processing: AWK's forte is processing text-based data. It can parse, filter, and transform data with ease, making it an ideal choice for data pipelines, ETL (Extract-Transform-Load) operations, or data migration tasks.
- Log analysis: AWK's text processing capabilities make it a great tool for analyzing log files, filtering out unnecessary data, and extracting insights from logs.
- API integration: AWK can be used to integrate with APIs that return text-based data, such as CSV or JSON. It can parse the data, transform it into a desired format, and then pass it on to other applications or services.
Real-World Use Cases: AWK in Action
Several real-world examples demonstrate AWK's value in backend development:
- Data aggregation: A company needs to aggregate data from multiple sources, including CSV files, JSON APIs, and log files. AWK can be used to process these different data sources, extract relevant information, and combine it into a single, unified dataset.
- Log monitoring: A web application generates large log files that need to be analyzed to identify trends, errors, and performance issues. AWK can be used to parse the log files, filter out unnecessary data, and extract insights that can be used to improve the application's performance and reliability.
- Data migration: A company needs to migrate data from an old database to a new one. AWK can be used to extract data from the old database, transform it into the required format, and then load it into the new database.
AWK vs. Other Languages: How Does it Stack Up?
So, how does AWK compare to other languages commonly used in backend development? Here are a few key differences:
- Performance: AWK is generally faster than languages like Python or Ruby, particularly when it comes to text processing and data manipulation.
- Memory usage: AWK is designed to be memory-efficient, making it a great choice for applications that need to process large datasets.
- Learning curve: AWK has a relatively simple syntax, making it easy to learn and use, even for developers without prior experience.
Best Practices for Using AWK in Backend Development
To get the most out of AWK in backend development, follow these best practices:
- Keep it simple: AWK is designed for simple, text-based processing tasks. Avoid using it for complex, computationally intensive tasks.
- Use it for data processing: AWK excels at data processing and manipulation. Use it for tasks like data aggregation, filtering, and transformation.
- Integrate with other tools: AWK can be used in conjunction with other tools and languages to create powerful data pipelines and workflows.
Mastering AWK for Backend Development: Unlocking the Power of Text Processing
AWK, a versatile and powerful text processing language, has been a cornerstone of Unix-based systems for decades. Despite its age, AWK remains a vital tool for backend developers, offering a unique combination of simplicity, flexibility, and performance. In this article, we'll delve deeper into the world of AWK, exploring its features, use cases, and best practices for backend development.
The Basics of AWK: A Primer
For those new to AWK, let's start with the basics. AWK is a domain-specific language (DSL) designed for text processing, created in the 1970s by Alfred Aho, Peter Weinberger, and Brian Kernighan (whose initials form the language's name). AWK's primary function is to search and manipulate text files, using a simple, yet powerful syntax.
An AWK program consists of a series of patterns and actions. Patterns are used to match specific lines or conditions within a text file, while actions are the commands executed when a pattern is matched. AWK's syntax is straightforward, with a focus on readability:
pattern { action }
For example, the following AWK program prints the first and last names of users from a file:
$1 ~ /^user/ { print $2, $3 }
Here, $1
represents the first field, and $2
and $3
represent the second and third fields, respectively. The pattern /^user/
matches lines starting with the string "user".
Data Processing with AWK: A Powerful Tool
AWK's strength lies in its ability to efficiently process large text files. Its streaming architecture allows AWK to handle files of any size, making it an ideal tool for data processing tasks. Some common data processing use cases for AWK include:
- Data filtering: AWK can selectively extract specific data from a file based on conditions, such as filtering out invalid records or selecting data within a specific range.
- Data transformation: AWK can perform data transformations, such as converting date formats, reformatting text, or aggregating data.
- Data aggregation: AWK can perform complex aggregations, such as grouping data by specific fields and calculating sums or averages.
Here's an example of using AWK to aggregate data:
# Calculate the total sales by region
NR == 1 { region = $1 }
NR > 1 && $1 != region {
print region, total
region = $1
total = $2
}
NR > 1 && $1 == region { total += $2 }
END { print region, total }
This program groups sales data by region and calculates the total sales for each region.
AWK and Backend Development: A Winning Combination
AWK can be a valuable tool for backend developers, particularly when working with large datasets or log files. Some use cases for AWK in backend development include:
- Log analysis: AWK can be used to parse and analyze log files, extracting relevant information such as IP addresses, timestamps, or error messages.
- Data validation: AWK can be used to validate data formats, such as checking for invalid or missing data in a file.
- Data integration: AWK can be used to integrate data from multiple sources, aggregating and transforming data as needed.
Here's an example of using AWK to analyze log files:
$5 ~ /^HTTP/ { print $1, $4, $5 }
This program extracts the IP address, date, and HTTP status code from a log file.
Best Practices for Using AWK: Tips and Tricks
While AWK is a powerful tool, there are some best practices to keep in mind when using it:
- Use meaningful variable names: AWK's syntax can be concise, but using meaningful variable names can improve readability and maintainability.
- Use comments: Comments can help explain complex AWK programs and make them easier to understand.
- Test and validate: Always test and validate AWK programs to ensure they produce the expected output.
Conclusion: AWK - The Unsung Hero of Backend Development
AWK may not be as well-known as other programming languages, but it remains a powerful tool for backend development. Its ability to quickly and efficiently process text-based data makes it an attractive choice for data-intensive applications. By understanding AWK's strengths and weaknesses, developers can harness its power to build faster, more efficient, and more scalable applications. Whether you're working on data pipelines, log analysis, or API integration, AWK is definitely worth considering.